react显示bpmn流程图并且高亮当前节点的插件以及使用方法
时间: 2024-02-01 08:14:25 浏览: 24
可以使用 `bpmn-js` 库来显示 BPMN 流程图,并且结合 `bpmn-js-highlight` 插件来高亮当前节点。
首先,你需要安装 `bpmn-js` 和 `bpmn-js-highlight` 两个库:
```
npm install bpmn-js bpmn-js-highlight
```
然后在你的 React 项目中引入它们:
```javascript
import BpmnModeler from 'bpmn-js/lib/Modeler';
import BpmnViewer from 'bpmn-js/lib/Viewer';
import 'bpmn-js/dist/assets/bpmn-font/css/bpmn.css';
import 'bpmn-js/dist/assets/diagram-js.css';
import 'bpmn-js-highlight/styles/highlight.css';
import bpmnHighlight from 'bpmn-js-highlight';
```
其中,`BpmnModeler` 是用于编辑 BPMN 流程图的组件,`BpmnViewer` 是用于显示 BPMN 流程图的组件。
接着在你的 React 组件中使用它们:
```javascript
import React, { useEffect, useRef } from 'react';
const BpmnViewerComponent = ({ xml, activeElementId }) => {
const containerRef = useRef(null);
const viewerRef = useRef(null);
useEffect(() => {
if (containerRef.current) {
viewerRef.current = new BpmnViewer({
container: containerRef.current,
additionalModules: [
bpmnHighlight
]
});
}
}, []);
useEffect(() => {
if (viewerRef.current && xml) {
viewerRef.current.importXML(xml, (err) => {
if (err) {
console.log('Error rendering BPMN', err);
} else {
const canvas = viewerRef.current.get('canvas');
const overlays = viewerRef.current.get('overlays');
canvas.zoom('fit-viewport');
if (activeElementId) {
const element = viewerRef.current.get('elementRegistry').get(activeElementId);
if (element) {
overlays.add(activeElementId, 'highlight');
overlays.remove({ type: 'highlight', id: activeElementId });
}
}
}
});
}
}, [xml, activeElementId]);
return (
<div className="bpmn-container" ref={containerRef}></div>
);
};
```
其中,`xml` 是 BPMN 流程图的 XML 字符串,`activeElementId` 是要高亮的节点的 ID。
在 `useEffect` 中,我们初始化了 `BpmnViewer` 并且将其实例存储在 `viewerRef` 中。然后在第二个 `useEffect` 中,我们使用 `viewerRef.current.importXML` 方法来加载 BPMN 流程图,并且根据 `activeElementId` 高亮指定的节点。
最后,我们在组件中使用 `BpmnViewerComponent` 来显示 BPMN 流程图:
```jsx
<BpmnViewerComponent xml={xml} activeElementId={activeElementId} />
```
其中,`xml` 和 `activeElementId` 是从组件的 props 中获取的。
相关推荐
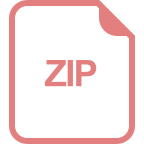
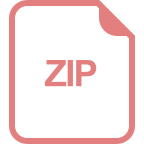














