我需要树莓派mpu6050的库
时间: 2023-10-07 13:07:56 浏览: 95
你可以使用Python的smbus库来与MPU6050通信。以下是使用smbus库的Python代码示例:
```python
import smbus
import math
import time
# MPU6050 Registers and their addresses
PWR_MGMT_1 = 0x6B
SMPLRT_DIV = 0x19
CONFIG = 0x1A
GYRO_CONFIG = 0x1B
INT_ENABLE = 0x38
ACCEL_XOUT_H = 0x3B
ACCEL_YOUT_H = 0x3D
ACCEL_ZOUT_H = 0x3F
TEMP_OUT_H = 0x41
GYRO_XOUT_H = 0x43
GYRO_YOUT_H = 0x45
GYRO_ZOUT_H = 0x47
bus = smbus.SMBus(1) # or 0 for older version of Raspberry Pi
DEVICE_ADDRESS = 0x68 # MPU6050 device address
def read_byte(reg):
return bus.read_byte_data(DEVICE_ADDRESS, reg)
def read_word(reg):
high = bus.read_byte_data(DEVICE_ADDRESS, reg)
low = bus.read_byte_data(DEVICE_ADDRESS, reg+1)
val = (high << 8) + low
return val
def read_word_2c(reg):
val = read_word(reg)
if (val & 0x8000):
return -((65535 - val) + 1)
else:
return val
def dist(a,b):
return math.sqrt((a*a)+(b*b))
def get_y_rotation(x,y,z):
radians = math.atan2(x, dist(y,z))
return -math.degrees(radians)
def get_x_rotation(x,y,z):
radians = math.atan2(y, dist(x,z))
return math.degrees(radians)
bus.write_byte_data(DEVICE_ADDRESS, SMPLRT_DIV, 0)
bus.write_byte_data(DEVICE_ADDRESS, PWR_MGMT_1, 1)
bus.write_byte_data(DEVICE_ADDRESS, CONFIG, 0)
bus.write_byte_data(DEVICE_ADDRESS, GYRO_CONFIG, 24)
bus.write_byte_data(DEVICE_ADDRESS, INT_ENABLE, 1)
time.sleep(0.1)
while True:
accel_xout = read_word_2c(ACCEL_XOUT_H)
accel_yout = read_word_2c(ACCEL_YOUT_H)
accel_zout = read_word_2c(ACCEL_ZOUT_H)
x_rotation = get_x_rotation(accel_xout, accel_yout, accel_zout)
gyro_xout = read_word_2c(GYRO_XOUT_H)
gyro_yout = read_word_2c(GYRO_YOUT_H)
gyro_zout = read_word_2c(GYRO_ZOUT_H)
print("gyro_xout: ", gyro_xout, " scaled: ", (gyro_xout / 131))
print("gyro_yout: ", gyro_yout, " scaled: ", (gyro_yout / 131))
print("gyro_zout: ", gyro_zout, " scaled: ", (gyro_zout / 131))
print("x rotation: ", get_x_rotation(accel_xout, accel_yout, accel_zout))
print("y rotation: ", get_y_rotation(accel_xout, accel_yout, accel_zout))
time.sleep(0.5)
```
请确保已经安装了smbus库,如果没有安装可以通过以下命令进行安装:
```
sudo apt-get install python-smbus
```
阅读全文
相关推荐
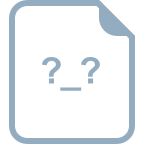
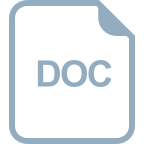


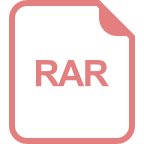




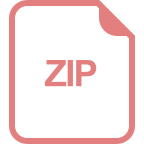
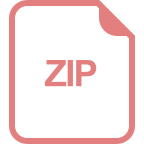




