void CreateSqList(SqList *&L,int a[],int n)和void CreateSqList(SqList *&L,int a[],int n)有什么区别
时间: 2024-01-24 10:18:53 浏览: 69
这两个函数看起来非常相似,但是它们的函数名是不同的,这意味着它们是两个不同的函数。
在第一个函数中,函数名为CreateSqList,参数包括一个指向SqList类型指针L、一个整型数组a和一个整型变量n。这个函数的作用是创建一个线性表,并将整型数组a中的前n个元素存储到线性表中。
在第二个函数中,函数名也是CreateSqList,参数同样包括一个指向SqList类型指针L、一个整型数组a和一个整型变量n。但是这个函数的作用是在已经存在的线性表L的基础上,将整型数组a中的前n个元素追加到线性表的末尾。
因此,这两个函数的主要区别在于它们的功能不同,第一个函数是创建一个新的线性表,第二个函数是向已有的线性表中添加元素。
相关问题
void CreateSqList(SqL *&L,int a[],int n)和void CreateSqList(SqList *&L,int a[],int n)有什么区别
这两个函数的区别在于参数类型中的一个符号“*”。它表示指针类型。
- `void CreateSqList(SqL *&L,int a[],int n)`中,参数 `L` 是指向 `SqL` 指针类型的指针,即 `L` 存储的是指向 `SqL` 类型数据的地址,函数执行完后,`L` 可能会指向新创建的 `SqL` 类型数据,因此使用引用传递。
- `void CreateSqList(SqList *&L,int a[],int n)`中,参数 `L` 是指向 `SqList` 类型数据的指针,即 `L` 存储的是指向 `SqList` 类型数据的地址,函数执行完后,`L` 可能会指向新创建的 `SqList` 类型数据,因此使用引用传递。
两个函数的区别在于它们创建的数据类型不同,一个是 `SqL` 类型,一个是 `SqList` 类型。同时,第一个函数传递的是指向指针类型数据的指针,第二个函数传递的是指向数据类型的指针。
构造一个顺序表,要求:顺序表的基本操作如下: (1)构造一个空的顺序表L,其基本操作为InitSqList (SqList *L)。 (2)顺序表L已存在,销毁顺序表L,其基本操作为DestroySqList(SqList *L)。 (3)顺序表L已存在,判断顺序表L是否为空表,若是则返回0,否则返回1,调用函数可通过判断函数返回值确定结果状态,其基本操作为SqListEmpty(SqList *L)。 (4)顺序表L已经存在,返回顺序表L中数据元素个数,其基本操作为SqListLength(SqList *L)。 (5)顺序表L已存在,依次输出顺序表L,其基本操作为DispSqList(SqList *L)。 (6)顺序表L已存在,返回顺序表L中第i个数据元素的值,其基本操作为GetSqListElem(SqList *L, int i, ElemType e)。 (7)顺序表L已存在,返回顺序表L中第1个与e的值相等的数据元素的位序,其基本操作为LocateSqListElem(SqList *L, ElemType e)。 (8)顺序表L已存在,在顺序表L中第i个位置上插入新的数据元素e,顺序表L的长度加1,其基本操作为SqListInsert(SqList *L, int i, ElemType e)。 (9)顺序表L已存在,删除顺序表L的第i个数据元素,顺序表L的长度减1,其基本操作为SqListDelete(SqList *L, int i)。 (10)根据给定数组中的数据,整体建立顺序表L,其基本操作为CreateSqList(SqList *L, ElemType a[ ], int n)
以下是一个基于动态内存分配的顺序表的实现,包含了题目要求的所有基本操作:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100 // 顺序表最大长度
typedef int ElemType; // 定义数据元素类型
typedef struct {
ElemType* data; // 存储数据的数组指针
int length; // 顺序表当前长度
int capacity; // 顺序表的容量
} SqList; // 顺序表结构体
// 初始化顺序表
void InitSqList(SqList* L) {
L->data = (ElemType*)malloc(MAXSIZE * sizeof(ElemType));
if (!L->data) {
printf("内存分配失败!\n");
exit(1);
}
L->length = 0;
L->capacity = MAXSIZE;
}
// 销毁顺序表
void DestroySqList(SqList* L) {
free(L->data);
L->data = NULL;
L->length = 0;
L->capacity = 0;
}
// 判断顺序表是否为空
int SqListEmpty(SqList* L) {
return L->length == 0;
}
// 返回顺序表长度
int SqListLength(SqList* L) {
return L->length;
}
// 输出顺序表
void DispSqList(SqList* L) {
if (SqListEmpty(L)) {
printf("顺序表为空!\n");
return;
}
printf("顺序表元素为:");
for (int i = 0; i < L->length; i++) {
printf("%d ", L->data[i]);
}
printf("\n");
}
// 获取指定位置的元素值
int GetSqListElem(SqList* L, int i, ElemType* e) {
if (i < 1 || i > L->length) {
printf("访问越界!\n");
return 0;
}
*e = L->data[i - 1];
return 1;
}
// 查找元素位置
int LocateSqListElem(SqList* L, ElemType e) {
for (int i = 0; i < L->length; i++) {
if (L->data[i] == e) {
return i + 1;
}
}
return 0;
}
// 插入元素
int SqListInsert(SqList* L, int i, ElemType e) {
if (i < 1 || i > L->length + 1) {
printf("插入位置错误!\n");
return 0;
}
if (L->length == L->capacity) {
printf("顺序表已满!\n");
return 0;
}
for (int j = L->length - 1; j >= i - 1; j--) {
L->data[j + 1] = L->data[j];
}
L->data[i - 1] = e;
L->length++;
return 1;
}
// 删除元素
int SqListDelete(SqList* L, int i) {
if (i < 1 || i > L->length) {
printf("删除位置错误!\n");
return 0;
}
for (int j = i; j < L->length; j++) {
L->data[j - 1] = L->data[j];
}
L->length--;
return 1;
}
// 创建顺序表
void CreateSqList(SqList* L, ElemType a[], int n) {
if (n > MAXSIZE) {
printf("数组长度超出最大值!\n");
exit(1);
}
for (int i = 0; i < n; i++) {
L->data[i] = a[i];
}
L->length = n;
}
int main() {
SqList L;
InitSqList(&L);
// 测试基本操作
printf("初始化顺序表:\n");
printf("顺序表的长度为:%d\n", SqListLength(&L));
printf("是否为空表:%d\n", SqListEmpty(&L));
DispSqList(&L);
printf("插入元素:\n");
SqListInsert(&L, 1, 1);
SqListInsert(&L, 2, 2);
SqListInsert(&L, 3, 3);
SqListInsert(&L, 4, 4);
DispSqList(&L);
printf("删除元素:\n");
SqListDelete(&L, 2);
DispSqList(&L);
printf("查找元素:\n");
ElemType e;
GetSqListElem(&L, 2, &e);
printf("第二个元素为:%d\n", e);
printf("元素6的位置为:%d\n", LocateSqListElem(&L, 6));
printf("创建顺序表:\n");
ElemType a[] = { 10, 20, 30, 40, 50 };
CreateSqList(&L, a, 5);
DispSqList(&L);
DestroySqList(&L);
return 0;
}
```
注意,在动态内存分配的情况下,需要在销毁顺序表时调用 `free` 函数释放分配的内存空间,以防止内存泄漏。
阅读全文
相关推荐
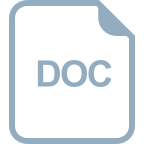
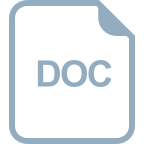
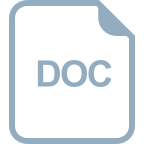
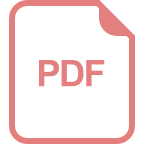
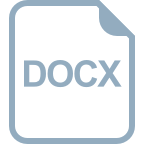
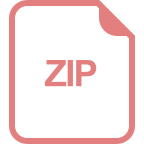
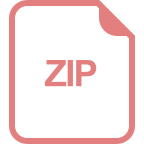
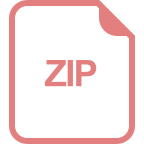
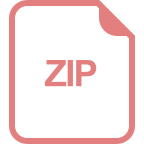
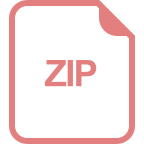
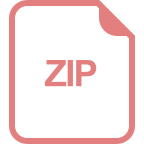
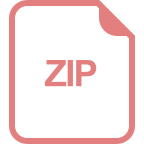