java圣诞树粒子特效代码
时间: 2024-12-20 19:11:08 浏览: 48
在Java中创建圣诞树粒子特效通常会涉及到使用动画、图形处理库(如Swing或JavaFX)以及时间驱动的更新循环。下面是一个简单的示例,展示如何使用JavaFX来实现基本的圣诞树形状和随机粒子效果:
```java
import javafx.animation.AnimationTimer;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class ChristmasTreeParticles {
private Canvas canvas;
private Stage stage;
private AnimationTimer timer;
public void init() {
stage = new Stage();
stage.setTitle("Christmas Tree Particle Effect");
stage.setWidth(800);
stage.setHeight(600);
// 创建画布
canvas = new Canvas(stage.getWidth(), stage.getHeight());
Scene scene = new Scene(canvas);
stage.setScene(scene);
// 圣诞树形状
Rectangle tree = new Rectangle(0, 0, 500, 700);
tree.setFill(Color.RED); // 红色背景
tree.setStroke(Color.WHITE);
scene.getChildren().add(tree);
// 初始化粒子
int particlesCount = 100;
for (int i = 0; i < particlesCount; i++) {
addParticle();
}
// 开始动画
timer = new AnimationTimer() {
@Override
public void handle(long now) {
updateParticles();
// 每秒更新一次
timer.schedule(this, 1000);
}
};
timer.start();
stage.show();
}
private void addParticle() {
// 添加随机位置和颜色的新粒子
CircleParticle particle = new CircleParticle(canvas.getGraphicsContext2D(),
Math.random() * stage.getWidth(),
Math.random() * stage.getHeight(),
Math.random() * 10,
Color.web("#" + Integer.toHexString((int)(Math.random()*16777215)))); // 随机颜色
canvas.getChildren().add(particle);
}
private void updateParticles() {
for (CircleParticle particle : canvas.getChildren()) {
if (particle.isOffScreen()) { // 当粒子离开屏幕时删除它
canvas.getChildren().remove(particle);
} else {
particle.update(); // 更新粒子的位置等属性
}
}
}
// 示例粒子类
static class CircleParticle extends Node {
// ... 实现粒子的生命周期管理,位置更新等
}
public static void main(String[] args) {
Platform.runLater(() -> {
new ChristmasTreeParticles().init();
});
}
}
```
阅读全文
相关推荐











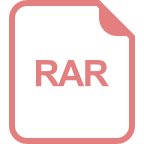
