vue3+ts+uni-app实现电子签名
时间: 2023-12-21 15:06:13 浏览: 54
根据提供的引用内容,我们可以使用uni-app的canvas组件来实现电子签名。以下是实现步骤:
1. 在uni-app项目中安装canvas组件:`npm install --save uni-canvas`
2. 在需要使用电子签名的页面中引入canvas组件:
```vue
<template>
<view>
<canvas :canvas-id="canvasId" @touchstart="handleTouchStart" @touchmove="handleTouchMove" @touchend="handleTouchEnd"></canvas>
<button @click="clearCanvas">清除</button>
<button @click="saveCanvas">保存</button>
</view>
</template>
<script>
import uniCanvas from 'uni-canvas';
export default {
components: {
uniCanvas
},
data() {
return {
canvasId: 'myCanvas',
ctx: null,
isDrawing: false,
lastX: 0,
lastY: 0
}
},
mounted() {
this.ctx = uni.createCanvasContext(this.canvasId, this);
},
methods: {
handleTouchStart(e) {
this.isDrawing = true;
this.lastX = e.touches[0].x;
this.lastY = e.touches[0].y;
},
handleTouchMove(e) {
if (!this.isDrawing) return;
this.ctx.beginPath();
this.ctx.moveTo(this.lastX, this.lastY);
this.ctx.lineTo(e.touches[0].x, e.touches[0].y);
this.ctx.stroke();
this.lastX = e.touches[0].x;
this.lastY = e.touches[0].y;
},
handleTouchEnd() {
this.isDrawing = false;
},
clearCanvas() {
this.ctx.clearRect(0, 0, uni.upx2px(375), uni.upx2px(500));
},
saveCanvas() {
uni.canvasToTempFilePath({
canvasId: this.canvasId,
success: function(res) {
uni.saveImageToPhotosAlbum({
filePath: res.tempFilePath,
success: function() {
uni.showToast({
title: '保存成功'
});
}
});
}
}, this);
}
}
}
```
在模板中,我们使用canvas组件来绘制电子签名,同时添加了两个按钮,一个用于清除画布,一个用于保存签名。在脚本中,我们使用uni.createCanvasContext方法获取canvas上下文,然后监听touch事件来绘制签名。最后,我们使用uni.canvasToTempFilePath方法将canvas转换为图片,并使用uni.saveImageToPhotosAlbum方法将图片保存到相册中。
3. 在tsconfig.json文件中添加以下配置:
```json
{
"compilerOptions": {
"target": "es6",
"module": "esnext",
"moduleResolution": "node",
"strict": true,
"jsx": "preserve",
"sourceMap": true,
"resolveJsonModule": true,
"esModuleInterop": true,
"lib": [
"esnext",
"dom",
"dom.iterable",
"scripthost"
]
},
"include": [
"src/**/*.ts",
"src/**/*.tsx",
"src/**/*.vue",
"tests/**/*.ts",
"tests/**/*.tsx"
],
"exclude": [
"node_modules"
]
}
```
在tsconfig.json文件中,我们将target设置为es6,module设置为esnext,同时开启了strict模式。
相关推荐
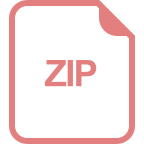
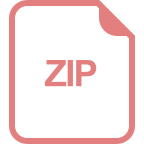
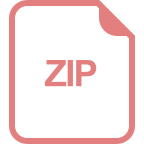














