输入判断字符串是否带字母python代码
时间: 2023-06-04 19:06:27 浏览: 108
可以使用正则表达式判断字符串中是否带有字母,示例代码如下:
```python
import re
def has_letter(s):
# 判断字符串中是否有字母
pattern = re.compile('[a-zA-Z]')
match = pattern.search(s)
if match:
return True
else:
return False
# 测试
s1 = 'Hello, World!'
s2 = '123456'
print(has_letter(s1)) # True
print(has_letter(s2)) # False
```
相关问题
python输入一个字符串判断字符串是否是对称字符串代码
以下是一个简单的Python代码,用于判断一个字符串是否是对称字符串:
```
def is_symmetric(string):
# 首先去除字符串中的空格,并将所有字符转换为小写字母
string = string.replace(" ", "").lower()
# 判断字符串是否是回文字符串
if string == string[::-1]:
return True
else:
return False
```
这个函数将输入的字符串中的所有空格去掉,并将所有字符转换为小写字母。然后,它将判断处理后的字符串是否等于其反向字符串。如果是,函数将返回True,否则返回False。
判断各种字符串是否合规python代码
以下是一个Python函数,可以判断各种字符串是否合规:
```python
def is_valid_string(s, min_length=1, max_length=None, allow_spaces=False, allow_digits=False,
allow_letters=False, allow_uppercase=False, allow_lowercase=False, allow_symbols=False,
allowed_symbols=None):
# 检查字符串长度是否合规
if max_length is not None and len(s) > max_length:
return False
if len(s) < min_length:
return False
# 检查字符串中是否只包含指定类型的字符
if not allow_spaces and " " in s:
return False
if not allow_digits and any(char.isdigit() for char in s):
return False
if not allow_letters and any(char.isalpha() for char in s):
return False
if not allow_uppercase and any(char.isupper() for char in s):
return False
if not allow_lowercase and any(char.islower() for char in s):
return False
if not allow_symbols and any(not char.isalnum() for char in s):
return False
# 检查字符串中是否只包含指定的符号
if allowed_symbols is not None and any(char not in allowed_symbols for char in s):
return False
return True
```
该函数接受一个字符串作为输入,并检查字符串是否合规。可以通过一些参数来定义字符串的合规条件,例如:
- `min_length`:字符串的最小长度,默认为1。
- `max_length`:字符串的最大长度,默认为None,表示不限制长度。
- `allow_spaces`:是否允许字符串中包含空格,默认为False。
- `allow_digits`:是否允许字符串中包含数字,默认为False。
- `allow_letters`:是否允许字符串中包含字母,默认为False。
- `allow_uppercase`:是否允许字符串中包含大写字母,默认为False。
- `allow_lowercase`:是否允许字符串中包含小写字母,默认为False。
- `allow_symbols`:是否允许字符串中包含符号,默认为False。
- `allowed_symbols`:允许的符号列表,只有在`allow_symbols`为True时才会生效,默认为None,表示不限制符号。
该函数返回一个布尔值,表示字符串是否合规。如果合规,返回True,否则返回False。
你可以使用该函数来检查各种字符串是否合规,例如:
```python
# 检查是否只包含数字
print(is_valid_string("12345", allow_digits=True)) # 输出 True
print(is_valid_string("1234a5", allow_digits=True)) # 输出 False
# 检查是否包含指定符号
print(is_valid_string("abc@def", allowed_symbols=["@", "."])) # 输出 True
print(is_valid_string("abc.def", allowed_symbols=["@", "."])) # 输出 False
```
阅读全文
相关推荐
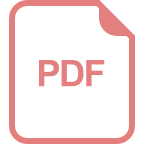
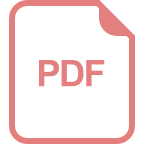













