如何让一个QLabel旋转(请使用c++)
时间: 2024-06-08 19:07:02 浏览: 280
你可以通过QPropertyAnimation动画类来实现QLabel的旋转,以下是一个简单的示例代码:
```c++
#include <QApplication>
#include <QLabel>
#include <QPropertyAnimation>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QLabel label("Hello, world!");
label.show();
// 创建一个旋转动画,将label从0度旋转到360度
QPropertyAnimation *animation = new QPropertyAnimation(&label, "rotation");
animation->setDuration(2000); // 设置动画时间为2秒
animation->setStartValue(0);
animation->setEndValue(360);
animation->setEasingCurve(QEasingCurve::InOutQuad); // 设置缓动函数,使动画更加自然
// 开始动画
animation->start();
return a.exec();
}
```
在上面的示例代码中,我们首先创建了一个QLabel,并将其显示出来。然后创建了一个QPropertyAnimation对象,将其关联到label的“rotation”属性上,设置动画时间、起始值、终止值和缓动函数。最后调用start()函数开始动画。当动画结束后,label会自动回到原来的位置。
需要注意的是,QLabel默认情况下是没有“rotation”属性的,因此我们需要通过Q_PROPERTY宏来为其定义一个属性。具体代码如下:
```c++
class RotatableLabel : public QLabel
{
Q_OBJECT
Q_PROPERTY(qreal rotation READ rotation WRITE setRotation)
public:
RotatableLabel(QWidget *parent = nullptr) : QLabel(parent) {}
qreal rotation() const { return m_rotation; }
void setRotation(qreal rotation) { m_rotation = rotation; update(); }
protected:
void paintEvent(QPaintEvent *event) override
{
QPainter painter(this);
painter.translate(width() / 2, height() / 2);
painter.rotate(m_rotation);
painter.translate(-width() / 2, -height() / 2);
QLabel::paintEvent(event);
}
private:
qreal m_rotation = 0;
};
```
在上面的代码中,我们定义了一个名为RotatableLabel的类,继承自QLabel,并且使用Q_PROPERTY宏为其定义了一个“rotation”属性。在paintEvent函数中,我们先将painter的坐标系移动到label的中心位置,然后旋转坐标系,最后再将坐标系移动回原来的位置,最后调用QLabel的paintEvent函数绘制label的内容。通过这样的方式,我们就可以在不改变QLabel本身的情况下旋转它了。
阅读全文
相关推荐

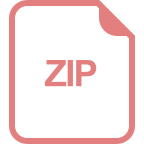
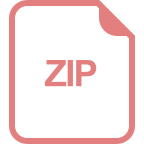
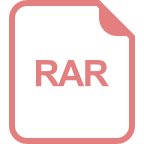
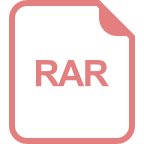
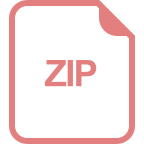
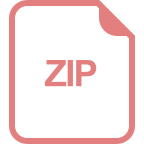
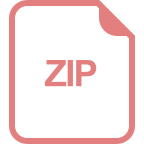
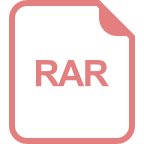
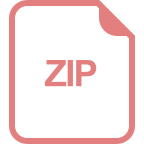
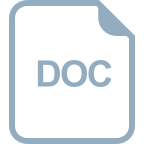
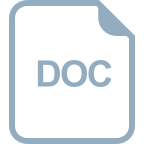



