使用懒汉单例模式和饿汉单例模式模拟产生本班的班长
时间: 2023-04-12 07:01:30 浏览: 121
可以使用懒汉单例模式和饿汉单例模式来模拟产生本班的班长。懒汉单例模式是在需要时才创建实例,而饿汉单例模式是在类加载时就创建实例。以下是懒汉单例模式和饿汉单例模式的示例代码:
懒汉单例模式:
```
public class ClassPresident {
private static ClassPresident instance;
private ClassPresident() {}
public static synchronized ClassPresident getInstance() {
if (instance == null) {
instance = new ClassPresident();
}
return instance;
}
}
```
饿汉单例模式:
```
public class ClassPresident {
private static ClassPresident instance = new ClassPresident();
private ClassPresident() {}
public static ClassPresident getInstance() {
return instance;
}
}
```
相关问题
使用懒汉单例模式和饿汉单例模式模拟产生本班的班长。
### 回答1:
可以使用以下代码实现懒汉单例模式:
```
public class ClassPresident {
private static ClassPresident instance;
private ClassPresident() {}
public static synchronized ClassPresident getInstance() {
if (instance == null) {
instance = new ClassPresident();
}
return instance;
}
}
```
可以使用以下代码实现饿汉单例模式:
```
public class ClassPresident {
private static final ClassPresident instance = new ClassPresident();
private ClassPresident() {}
public static ClassPresident getInstance() {
return instance;
}
}
```
### 回答2:
懒汉单例模式和饿汉单例模式都是常用的单例模式实现方式,下面将使用这两种方式来模拟产生本班的班长。
懒汉单例模式中,班长的实例会在第一次被调用时创建。我们可以定义一个班长类,其中只有一个私有的静态班长实例和一个公有的获取班长实例的方法。在此方法中,如果班长实例为空,则实例化一个班长对象,然后返回该实例。这样就保证了只有一个班长对象被创建,通过调用该方法可以获取到这个班长对象。
饿汉单例模式中,班长的实例会在类加载时就创建好。我们可以在班长类的静态成员中直接创建一个班长实例,并且定义一个公有的获取班长实例的方法,该方法直接返回这个已经创建好的班长实例。这样在调用这个方法时,每次都可以获取到同一个班长对象。
懒汉单例模式和饿汉单例模式的区别在于实例的创建时间不同,一个是第一次使用时创建,一个是类加载时就创建。在本班的班长模拟中,我们可以选择使用任何一种方式来实现。无论是懒汉单例模式还是饿汉单例模式,我们只需要保证只有一个班长实例被创建即可。
总结起来,使用懒汉单例模式和饿汉单例模式模拟产生本班的班长都可以实现,只需要保证只有一个班长对象被创建并且可以通过公有的方法获取到这个对象即可。
### 回答3:
懒汉单例模式和饿汉单例模式都可以用来模拟产生本班的班长,下面分别进行介绍。
懒汉单例模式是指在需要的时候才会创建实例,不会提前初始化。对于班长这个角色来说,班级中的学生先进行投票选举,并不会提前确定班长人选。因此,可以使用懒汉单例模式来模拟班长的产生。懒汉单例模式的实现方式可以是在获取班长的实例时,先判断是否已经存在班长实例,如果不存在则进行选举产生一个班长,并保存实例,以便下次获取。这样每次获取班长实例的时候都可以保证只有一个实例被创建和使用,符合单例模式的特点。
相比之下,饿汉单例模式是在类加载时就创建并初始化实例,无论是否需要都会提前初始化。在模拟班长的例子中,饿汉单例模式可以是在开学之初就确定一个学生作为班长,无需投票选举。这样在获取班长实例时,直接返回之前已经初始化好的班长实例即可。饿汉单例模式的实现方式是在类加载时候使用私有的静态成员变量来直接初始化唯一的班长实例,因此可以保证只有一个实例被创建和使用。
总结来说,使用懒汉单例模式模拟产生本班的班长,可以在需要时进行选举产生一个班长实例,并保存实例以备后续使用;而使用饿汉单例模式来模拟产生本班的班长,则是在开学之初确定一个班长,并在需要时直接返回该实例。
使用懒汉单例模式和饿汉单例模式模拟产生本班的班长,并写出客户端代码
好的,我可以回答这个问题。以下是使用懒汉单例模式和饿汉单例模式模拟产生本班的班长的代码:
懒汉单例模式:
```java
public class ClassLeader {
private static ClassLeader instance;
private String name;
private ClassLeader(String name) {
this.name = name;
}
public static synchronized ClassLeader getInstance(String name) {
if (instance == null) {
instance = new ClassLeader(name);
}
return instance;
}
public void introduce() {
System.out.println("我是本班的班长,我的名字是" + name);
}
}
```
饿汉单例模式:
```java
public class ClassLeader {
private static ClassLeader instance = new ClassLeader("张三");
private String name;
private ClassLeader(String name) {
this.name = name;
}
public static ClassLeader getInstance() {
return instance;
}
public void introduce() {
System.out.println("我是本班的班长,我的名字是" + name);
}
}
```
客户端代码:
```java
public class Client {
public static void main(String[] args) {
// 使用懒汉单例模式
ClassLeader leader1 = ClassLeader.getInstance("李四");
leader1.introduce();
ClassLeader leader2 = ClassLeader.getInstance("王五");
leader2.introduce();
// 使用饿汉单例模式
ClassLeader leader3 = ClassLeader.getInstance();
leader3.introduce();
}
}
```
希望这个回答能够帮到你!
相关推荐
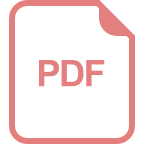
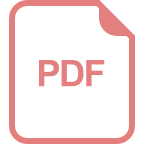





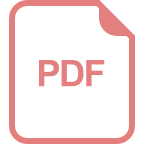
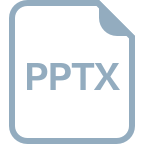
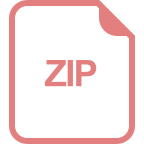