给我一个adc输入然后dac输出的keil程序
时间: 2024-05-04 08:18:07 浏览: 24
以下是一个基本的 ADC 和 DAC 的代码示例,使用 Keil µVision IDE 编写,将 ADC 转换的值通过 DAC 输出。这个例子使用了 STM32F4xx MCU。
```c
#include "stm32f4xx.h"
#define ADC1_DR_ADDRESS ((uint32_t)0x4001204C)
#define DAC_DHR12R1_ADDRESS ((uint32_t)0x40007408)
void ADC_Config(void);
void DAC_Config(void);
int main(void) {
uint16_t ADCValue;
ADC_Config();
DAC_Config();
while (1) {
ADC_SoftwareStartConv(ADC1);
while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET);
ADCValue = ADC_GetConversionValue(ADC1);
DAC_SetChannel1Data(DAC_Align_12b_R, ADCValue);
}
}
void ADC_Config(void) {
GPIO_InitTypeDef GPIO_InitStructure;
ADC_InitTypeDef ADC_InitStructure;
ADC_CommonInitTypeDef ADC_CommonInitStructure;
/* Enable GPIOA clock */
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
/* Configure ADC1 Channel 0 pin as analog input */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* Enable the HSI oscillator */
RCC_HSICmd(ENABLE);
/* Wait until the HSI oscillator is ready */
while (RCC_GetFlagStatus(RCC_FLAG_HSIRDY) == RESET);
/* Configure the ADC clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
/* ADC Common Init */
ADC_CommonInitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_CommonInitStructure.ADC_Prescaler = ADC_Prescaler_Div2;
ADC_CommonInitStructure.ADC_DMAAccessMode = ADC_DMAAccessMode_Disabled;
ADC_CommonInitStructure.ADC_TwoSamplingDelay = ADC_TwoSamplingDelay_5Cycles;
ADC_CommonInit(&ADC_CommonInitStructure);
/* ADC1 Init */
ADC_InitStructure.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = DISABLE;
ADC_InitStructure.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStructure);
/* ADC1 Regular Channel Config */
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_3Cycles);
/* Enable ADC1 */
ADC_Cmd(ADC1, ENABLE);
}
void DAC_Config(void) {
GPIO_InitTypeDef GPIO_InitStructure;
DAC_InitTypeDef DAC_InitStructure;
/* Enable GPIOA clock */
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
/* Configure PA.04 (DAC1_OUT1) as analog output */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* Enable DAC clock */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_DAC, ENABLE);
/* DAC channel1 Configuration */
DAC_InitStructure.DAC_Trigger = DAC_Trigger_None;
DAC_InitStructure.DAC_OutputBuffer = DAC_OutputBuffer_Enable;
DAC_Init(DAC_Channel_1, &DAC_InitStructure);
/* Enable DAC Channel1 */
DAC_Cmd(DAC_Channel_1, ENABLE);
/* Set DAC Channel1 DHR12R register */
DAC_SetChannel1Data(DAC_Align_12b_R, 0x0000);
}
```
这个代码将 ADC1 配置为单次转换模式,使用 PA0 作为 ADC 输入通道。DAC1 用于输出,使能 GPIOA4 作为模拟输出。然后在 while 循环中,读取 ADC1 转换值并将其写入 DAC1 输出寄存器。这个例子可以根据需要进行修改,以适应特定的应用场景。
相关推荐
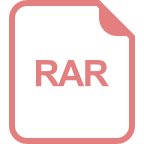
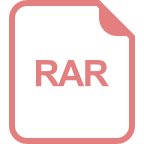
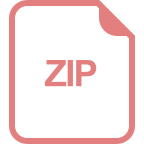















