vue高德地图自定义标记
时间: 2023-11-05 09:04:23 浏览: 88
Vue高德地图自定义标记可以通过以下步骤实现:
1. 在Vue组件中引入高德地图API:
```
import AMapLoader from '@amap/amap-jsapi-loader';
```
2. 在Vue组件中定义地图容器,并在mounted钩子函数中加载地图:
```
<template>
<div id="map-container"></div>
</template>
<script>
export default {
data() {
return {
map: null,
};
},
mounted() {
AMapLoader.load({
key: 'your-api-key',
version: '2.0',
plugins: ['AMap.Marker'],
}).then(() => {
this.initMap();
});
},
methods: {
initMap() {
this.map = new AMap.Map('map-container', {
center: [116.397428, 39.90923],
zoom: 13,
});
},
},
};
</script>
```
3. 在Vue组件中定义自定义标记的样式,并使用AMap.Marker类创建自定义标记对象:
```
<template>
<div id="map-container"></div>
</template>
<script>
export default {
data() {
return {
map: null,
markerStyle: {
width: '24px',
height: '24px',
background: 'url("your-marker-icon.png") no-repeat',
backgroundSize: '100% 100%',
},
};
},
mounted() {
AMapLoader.load({
key: 'your-api-key',
version: '2.0',
plugins: ['AMap.Marker'],
}).then(() => {
this.initMap();
this.addMarker();
});
},
methods: {
initMap() {
this.map = new AMap.Map('map-container', {
center: [116.397428, 39.90923],
zoom: 13,
});
},
addMarker() {
const marker = new AMap.Marker({
position: [116.397428, 39.90923],
map: this.map,
icon: new AMap.Icon(this.markerStyle),
});
},
},
};
</script>
```
4. 根据需要,可以在自定义标记上添加事件监听器:
```
<template>
<div id="map-container"></div>
</template>
<script>
export default {
data() {
return {
map: null,
markerStyle: {
width: '24px',
height: '24px',
background: 'url("your-marker-icon.png") no-repeat',
backgroundSize: '100% 100%',
},
};
},
mounted() {
AMapLoader.load({
key: 'your-api-key',
version: '2.0',
plugins: ['AMap.Marker'],
}).then(() => {
this.initMap();
this.addMarker();
});
},
methods: {
initMap() {
this.map = new AMap.Map('map-container', {
center: [116.397428, 39.90923],
zoom: 13,
});
},
addMarker() {
const marker = new AMap.Marker({
position: [116.397428, 39.90923],
map: this.map,
icon: new AMap.Icon(this.markerStyle),
});
marker.on('click', () => {
alert('Marker clicked!');
});
},
},
};
</script>
```
以上就是Vue高德地图自定义标记的实现方法。需要注意的是,自定义标记的样式需要根据实际情况进行调整。
阅读全文
相关推荐
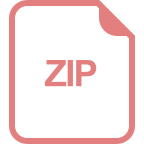
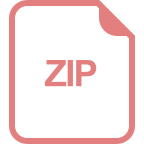

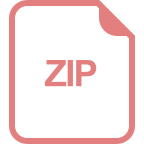
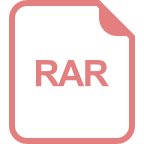
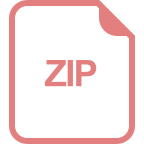
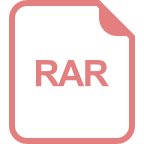










