<dlfcn.h> 编译
时间: 2023-09-15 16:23:03 浏览: 331
<fcn.h> 是一个头文件,用于在C和C++程序中使用动态链接库。在编译时,需要将-l dl选项添加到GCC命令中,以指定需要链接动态链接库。例如,如果您的程序需要链接libdl.so,编译命令应如下所示:
```
gcc -o program program.c -ldl
```
其中,program.c是您的程序源代码文件,-ldl选项告诉GCC将libdl.so链接到您的程序中。
相关问题
In file included from ../sysdeps/x86_64/nptl/tls.h:23, from ../include/link.h:51, from ../include/dlfcn.h:4, from ../sysdeps/generic/ldsodefs.h:32, from ../sysdeps/x86/ldsodefs.h:65, from ../sysdeps/gnu/ldsodefs.h:46, from ../sysdeps/unix/sysv/linux/ldsodefs.h:25, from ../sysdeps/x86/dl-get-cpu-features.c:19: ../sysdeps/unix/sysv/linux/x86/include/asm/prctl.h:5:15: fatal error: asm/prctl.h: No such file or directory 5 | #include_next <asm/prctl.h> | ^~~~~~~~~~~~~ compilation terminated. /glibc-2.35/build/sysd-rules:739: recipe for target '/glibc-2.35/build/elf/dl-get-cpu-features.os' failed make[2]: *** [/glibc-2.35/build/elf/dl-get-cpu-features.os] Error 1 make[2]: Leaving directory '/glibc-2.35/elf' Makefile:483: recipe for target 'elf/subdir_lib' failed make[1]: *** [elf/subdir_lib] Error 2 make[1]: Leaving directory '/glibc-2.35' Makefile:9: recipe for target 'all' failed make: *** [all] Error 2
这个错误提示表明编译 Glibc 时缺少 asm/prctl.h 文件。这个文件是 Linux 内核源代码中的一个头文件,需要安装 Linux 内核头文件才能解决该问题。
你可以使用以下命令来安装 Linux 内核头文件:
```
sudo apt-get install linux-headers-$(uname -r)
```
如果你运行的是其他 Linux 发行版,请使用相应的命令安装相应的内核头文件。安装完成后,重新编译 Glibc 库即可。
另外,如果你尝试使用 Glibc 的最新版本编译代码,建议使用较新的 Linux 内核版本,否则可能会遇到其他的编译错误。
//创建a.h、a.c,、b.h、b.c四个文件, //在a文件中创建求阶乘函数并实现, //在b文件中创建数组的排序函数并实现,要求将a,b编译成动态库文件。 //并编写test.c文件,引入a和b两个文件的功能并编译运行。
首先,我们创建 `a.h` 文件,其中包含阶乘函数的声明:
```c
#ifndef A_H
#define A_H
int factorial(int n);
#endif
```
然后,我们创建 `a.c` 文件,实现阶乘函数:
```c
#include "a.h"
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
```
接下来,我们创建 `b.h` 文件,其中包含数组排序函数的声明:
```c
#ifndef B_H
#define B_H
void sortArray(int arr[], int size);
#endif
```
然后,我们创建 `b.c` 文件,实现数组排序函数:
```c
#include "b.h"
void sortArray(int arr[], int size) {
int i, j, temp;
for (i = 0; i < size - 1; i++) {
for (j = 0; j < size - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
接下来,我们将 `a.c` 和 `b.c` 编译为动态库文件。在命令行中执行以下命令(假设您使用的是 GCC 编译器):
```shell
gcc -shared -o liba.so a.c
gcc -shared -o libb.so b.c
```
这将分别生成 `liba.so` 和 `libb.so` 两个动态库文件。
最后,我们编写 `test.c` 文件来测试引入的功能:
```c
#include <stdio.h>
#include <dlfcn.h> // 引入动态库相关的头文件
#include "a.h"
#include "b.h"
int main() {
// 加载动态库
void* liba_handle = dlopen("./liba.so", RTLD_LAZY);
void* libb_handle = dlopen("./libb.so", RTLD_LAZY);
if (!liba_handle || !libb_handle) {
printf("Failed to load dynamic libraries.\n");
return 1;
}
// 获取动态库中的函数指针
int (*factorial_ptr)(int) = dlsym(liba_handle, "factorial");
void (*sortArray_ptr)(int[], int) = dlsym(libb_handle, "sortArray");
if (!factorial_ptr || !sortArray_ptr) {
printf("Failed to get function pointers.\n");
return 1;
}
// 使用动态库中的函数
int result = factorial_ptr(5);
printf("Factorial of 5 is: %d\n", result);
int arr[] = {4, 2, 7, 1, 9};
int size = sizeof(arr) / sizeof(arr[0]);
sortArray_ptr(arr, size);
printf("Sorted array: ");
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// 关闭动态库
dlclose(liba_handle);
dlclose(libb_handle);
return 0;
}
```
请确保将 `test.c` 文件和生成的动态库文件(`liba.so` 和 `libb.so`)放在同一个目录下。在命令行中执行以下命令编译并运行 `test.c` 文件:
```shell
gcc -o test test.c -ldl
./test
```
这将编译并运行 `test.c` 文件,输出阶乘结果和排序后的数组。
阅读全文
相关推荐
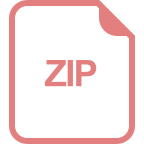
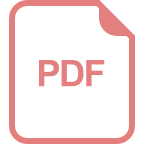
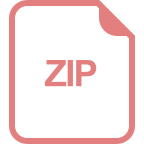
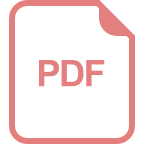
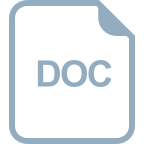
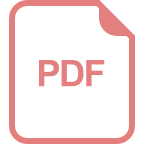
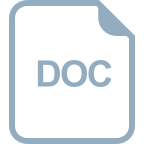
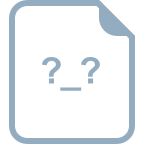
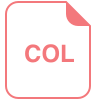





