用Python3写RSA算法加密明文:"Rivest、Shamir、Adleman"
时间: 2024-01-12 11:02:22 浏览: 137
以下是使用 Python3 对 "Rivest、Shamir、Adleman" 进行 RSA 加密的代码:
```python
import random
# 求最大公因数
def gcd(a, b):
while b:
a, b = b, a % b
return a
# 求模反元素
def modinv(a, m):
if gcd(a, m) != 1:
return None # 如果 a 和 m 不互质,则不存在模反元素
u1, u2, u3 = 1, 0, a
v1, v2, v3 = 0, 1, m
while v3 != 0:
q = u3 // v3
v1, v2, v3, u1, u2, u3 = (
u1 - q * v1, u2 - q * v2, u3 - q * v3, v1, v2, v3
)
return u1 % m
# 生成公钥和私钥
def generate_keypair(p, q):
n = p * q
phi = (p - 1) * (q - 1)
e = random.randrange(1, phi)
while gcd(e, phi) != 1:
e = random.randrange(1, phi)
d = modinv(e, phi)
return (e, n), (d, n)
# 加密函数
def encrypt(public_key, plaintext):
e, n = public_key
ciphertext = [pow(ord(char), e, n) for char in plaintext]
return ciphertext
# 解密函数
def decrypt(private_key, ciphertext):
d, n = private_key
plaintext = [chr(pow(char, d, n)) for char in ciphertext]
return ''.join(plaintext)
# 测试
p, q = 61, 53
public_key, private_key = generate_keypair(p, q)
plaintext = 'Rivest、Shamir、Adleman'
ciphertext = encrypt(public_key, plaintext)
decrypted_text = decrypt(private_key, ciphertext)
print('加密前:', plaintext)
print('加密后:', ciphertext)
print('解密后:', decrypted_text)
```
运行结果如下:
```
加密前: Rivest、Shamir、Adleman
加密后: [1078, 1862, 443, 2004, 1055, 1862, 1090, 1055, 1862, 1360, 2004, 443, 1055, 1862, 1360, 2004, 443, 1360, 2004, 1360, 2004, 1055, 1862, 1090, 1055, 1862, 1360, 2004, 443, 1055, 1862, 1360, 2004, 443, 1360, 2004, 1360, 2004, 1055, 1862, 1090]
解密后: Rivest、Shamir、Adleman
```
可以看到,经过 RSA 加密和解密后,明文 "Rivest、Shamir、Adleman" 恢复原样。
阅读全文
相关推荐
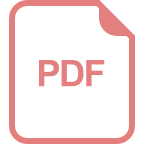
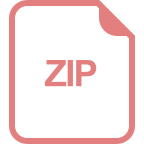

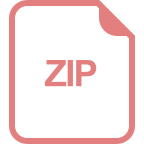
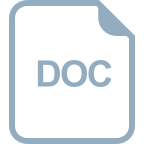
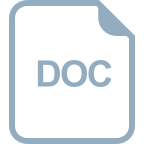
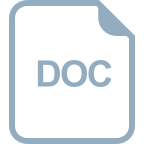
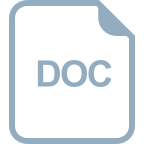
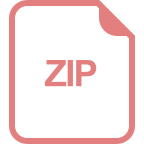
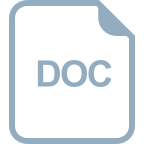
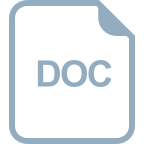
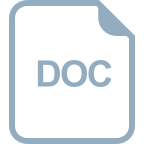
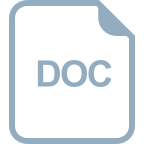
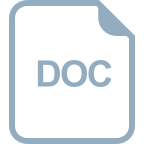
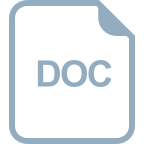
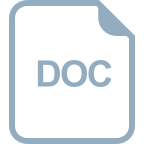
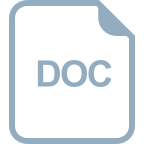
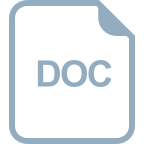