js如果实现歌词的滚动效果
时间: 2023-11-27 22:03:43 浏览: 141
要实现歌词的滚动效果,可以通过以下步骤:
1. 将歌词文本按照时间轴分割成多个段落,每个段落对应一句歌词。
2. 在页面中创建一个容器,用来显示当前正在播放的歌词。
3. 使用 JavaScript 监听音乐播放的时间轴,每当音乐时间轴与某个歌词段落的时间范围重合时,将该歌词段落显示在容器中。
4. 实现歌词滚动的效果,可以使用 CSS 动画或 JavaScript 操作 DOM 的方式,将当前歌词段落滚动到容器的中央或者上方,并且将已经显示完的歌词段落从容器中移除。
以下是一个简单的 JavaScript 实现示例:
```html
<div id="lyrics-container"></div>
<script>
const lyrics = [
{ time: 0, text: "Verse 1" },
{ time: 10, text: "I'm looking at an empty screen" },
{ time: 15, text: "Everything is quiet, not a thing to be seen" },
{ time: 22, text: "Suddenly a message pops up on my phone" },
{ time: 30, text: "It's you, I'm glad you're not alone" },
{ time: 36, text: "Chorus" },
{ time: 40, text: "We're all connected, even when we're far apart" },
{ time: 47, text: "Our hearts beat as one, it's a symphony of the heart" },
{ time: 54, text: "We're all connected, even when we're miles away" },
{ time: 61, text: "Our love is like a river, it flows stronger every day" },
{ time: 68, text: "Verse 2" },
{ time: 72, text: "I'm sitting in a crowded room" },
{ time: 77, text: "People are talking, but I'm feeling the gloom" },
{ time: 84, text: "Suddenly I hear a voice that sounds like home" },
{ time: 92, text: "It's you, I'm glad you're not alone" },
{ time: 98, text: "Chorus" },
{ time: 102, text: "We're all connected, even when we're far apart" },
{ time: 109, text: "Our hearts beat as one, it's a symphony of the heart" },
{ time: 116, text: "We're all connected, even when we're miles away" },
{ time: 123, text: "Our love is like a river, it flows stronger every day" },
];
const container = document.getElementById("lyrics-container");
// 当播放时间发生改变时触发的事件
const onTimeUpdate = (event) => {
const currentTime = event.target.currentTime;
// 查找当前时间对应的歌词段落
const currentLyric = lyrics.find((lyric) => {
return lyric.time <= currentTime && lyric.time + 5 > currentTime;
});
// 如果找到了歌词段落,则更新容器中的内容
if (currentLyric) {
container.innerHTML = currentLyric.text;
}
};
// 监听音乐播放时间的变化
document.getElementById("music-player").addEventListener("timeupdate", onTimeUpdate);
</script>
```
这个示例中,我们将歌词分割成多个对象,每个对象有一个时间属性和一个文本属性,用来表示当前时间段的歌词内容。在页面中创建了一个用来显示歌词的容器,然后使用 `addEventListener` 方法监听音乐播放时间的变化,每当时间发生改变时,就查找当前时间对应的歌词段落,并将其显示在容器中。具体的歌词滚动效果可以根据实际需求进行修改。
阅读全文
相关推荐
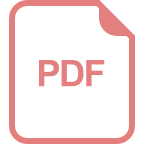
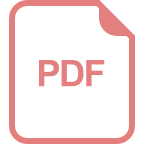
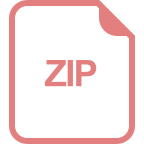
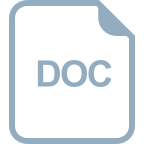
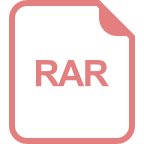
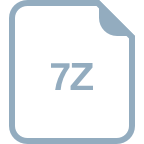
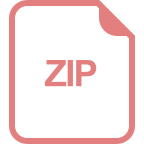
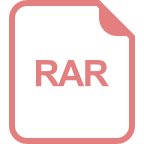
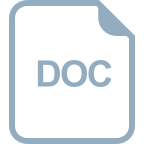
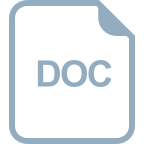
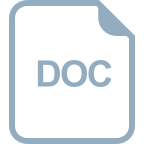

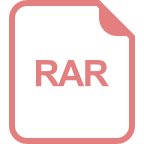
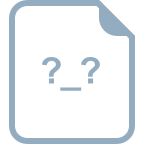
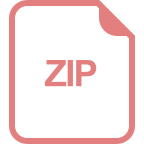
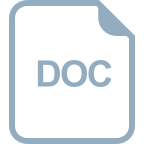
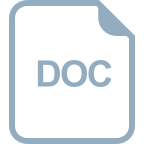