类型“HTMLElement | null”的参数不能赋给类型“HTMLDivElement | HTMLCanvasElement”的参数。 不能将类型“null”分配给类型“HTMLDivElement | HTMLCanvasElement”。ts(2345) const chartContainer: HTMLElement | null
时间: 2024-04-08 08:30:01 浏览: 35
出现这个错误是因为 `getElementById` 方法的返回类型是 `HTMLElement | null`,而 `echarts.init` 方法需要传入一个 `HTMLDivElement` 或 `HTMLCanvasElement` 类型的参数。
为了解决这个问题,你可以使用类型断言将返回值转为 `HTMLDivElement` 或 `HTMLCanvasElement` 类型。例如:
```typescript
const chartContainer = document.getElementById('chart-container') as HTMLDivElement;
```
或者,你可以使用可选链操作符(optional chaining)来确保获取到元素后再调用 `echarts.init` 方法。例如:
```typescript
const chartContainer = document.getElementById('chart-container');
if (chartContainer) {
const chart = echarts.init(chartContainer);
// 其他代码...
}
```
以上两种方式都可以解决类型不匹配的问题,你可以根据自己的喜好选择其中一种方式来处理。
相关问题
类型“HTMLElement | null”的参数不能赋给类型“HTMLElement”的参数。 不能将类型“null”分配给类型“HTMLElement”。
这个错误是因为 TypeScript 不确定 `document.getElementById` 方法返回的结果是否为 `null`,因此需要我们自己判断并处理这种情况。
可以使用非空断言操作符 `!` 来告诉 TypeScript,我们确定这个结果不会是 `null`。代码示例如下:
```typescript
const refreshInfoElement = document.getElementById('refresh-info')!;
```
这样做的前提是我们确定 `refresh-info` 这个元素一定会存在于 HTML 中。如果不能确定,可以使用可选链操作符 `?.` 来避免这个错误:
```typescript
const refreshInfoElement = document.getElementById('refresh-info');
if (refreshInfoElement) {
const refreshInfo = new RefreshInfo(refreshInfoElement);
refreshInfo.refresh();
window.onbeforeunload = () => {
refreshInfo.refresh();
};
}
```
这样做的好处是可以避免 `null` 引用错误,但需要在代码中加入判断逻辑,稍微复杂一些。
Type 'HTMLElement | null' is not assignable to type 'HTMLElement | undefined'. Type 'null' is not assignable to type 'HTMLElement | undefined'.
这个错误的意思是,你尝试将一个值类型为 `null` 的变量赋值给一个类型为 `HTMLElement | undefined` 的变量,但是 `null` 不属于 `HTMLElement` 或者 `undefined` 类型,所以会报错。
解决方法可以有两种:
1. 将类型改为 `HTMLElement | null`。这样就可以将 `null` 赋值给变量了。
2. 在赋值之前,先判断该变量是否为 `null`,如果是的话,就将其转换为 `undefined`。例如:
```typescript
let element: HTMLElement | undefined;
let nullableElement: HTMLElement | null = null;
if (nullableElement !== null) {
element = nullableElement; // 这里不会报错
}
```
相关推荐
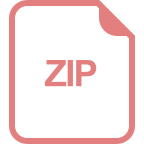
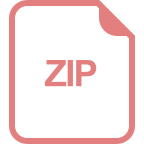












