springboot 导出word带表格和图片
时间: 2023-09-06 18:03:55 浏览: 285
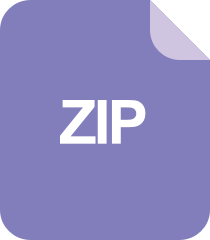
SpringBoot使用Word导出表格
在Spring Boot中使用Apache POI库可以实现导出带有表格和图片的Word文档。以下是一种实现方式的简述:
1. 首先,在pom.xml文件中添加Apache POI和Apache POI-ooxml的依赖项:
```xml
<dependencies>
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.17</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.17</version>
</dependency>
</dependencies>
```
2. 创建一个Java类,用于生成带有表格和图片的Word文档。可以使用以下代码作为参考:
```java
import org.apache.poi.xwpf.usermodel.*;
import java.io.FileOutputStream;
import java.io.IOException;
public class WordGenerator {
public void generateWord() throws IOException {
// 创建一个新的Word文档
XWPFDocument document = new XWPFDocument();
// 创建一个段落
XWPFParagraph paragraph = document.createParagraph();
// 创建一个表格
XWPFTable table = document.createTable(3, 2);
// 在表格中添加数据
table.getRow(0).getCell(0).setText("表格标题");
table.getRow(1).getCell(0).setText("数据1");
table.getRow(1).getCell(1).setText("数据2");
table.getRow(2).getCell(0).setText("数据3");
table.getRow(2).getCell(1).setText("数据4");
// 添加图片
XWPFParagraph imageParagraph = document.createParagraph();
XWPFRun imageRun = imageParagraph.createRun();
String imagePath = "图片路径";
int imageFormat = XWPFDocument.PICTURE_TYPE_PNG;
imageRun.addPicture(new FileInputStream(imagePath), imageFormat, "图片描述", Units.toEMU(200), Units.toEMU(200)); // 根据需要调整图片大小
// 保存文档
String outputPath = "输出路径";
FileOutputStream out = new FileOutputStream(outputPath);
document.write(out);
out.close();
System.out.println("Word文档生成成功!");
}
}
```
3. 在Spring Boot应用程序中调用`generateWord()`方法,即可生成带有表格和图片的Word文档。
请注意更换代码中的图片路径和输出路径为实际需要的路径,并根据需要进行调整。
阅读全文
相关推荐







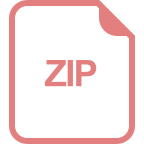
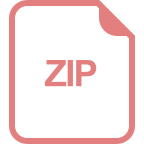
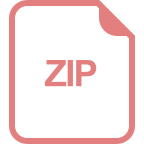
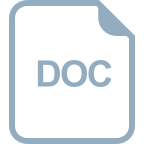
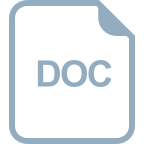
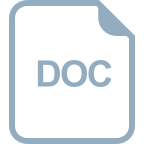
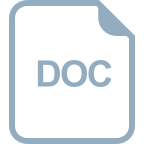


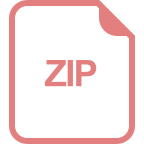