基于JDBC Java程序连接postgresql在StudentInfo表中插入,更新和删除一条记录,并将表中的记录在控制台中输出,使用PreparedStatement实现。
时间: 2024-03-23 21:43:12 浏览: 54
好的,以下是基于JDBC Java程序连接 PostgreSQL,在 StudentInfo 表中插入、更新和删除一条记录,并将表中的记录在控制台中输出的代码,使用 PreparedStatement 实现:
```java
import java.sql.*;
public class JDBCExample {
static final String JDBC_DRIVER = "org.postgresql.Driver";
static final String DB_URL = "jdbc:postgresql://localhost:5432/mydatabase";
static final String USER = "postgres";
static final String PASS = "mysecretpassword";
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt = null;
try {
// 注册 JDBC 驱动器
Class.forName(JDBC_DRIVER);
// 打开一个连接
System.out.println("连接数据库...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// 插入一条记录
System.out.println("插入一条记录到 StudentInfo 表...");
String sql = "INSERT INTO StudentInfo (id, name, age) VALUES (?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, 1);
stmt.setString(2, "张三");
stmt.setInt(3, 20);
stmt.executeUpdate();
// 更新一条记录
System.out.println("更新一条记录到 StudentInfo 表...");
sql = "UPDATE StudentInfo SET age = ? WHERE id = ?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, 21);
stmt.setInt(2, 1);
stmt.executeUpdate();
// 删除一条记录
System.out.println("删除一条记录从 StudentInfo 表...");
sql = "DELETE FROM StudentInfo WHERE id = ?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, 1);
stmt.executeUpdate();
// 输出表中所有记录
System.out.println("输出 StudentInfo 表中所有记录...");
sql = "SELECT id, name, age FROM StudentInfo";
stmt = conn.prepareStatement(sql);
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
// 关闭资源
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
System.out.println("Goodbye!");
}
}
}
```
注意,在运行代码之前,请确保已经安装了 PostgreSQL 数据库,并在其中创建了名为 `mydatabase` 的数据库,以及名为 `StudentInfo` 的表,并且表中已经包含了至少一条记录。同时,也需要将代码中的 `USER` 和 `PASS` 替换为你自己的数据库用户名和密码。
阅读全文
相关推荐
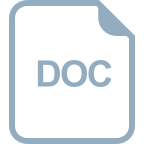
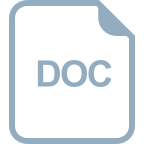
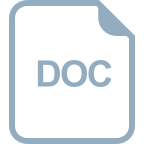

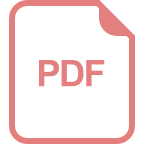
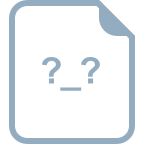
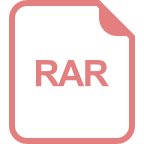
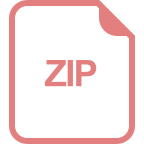
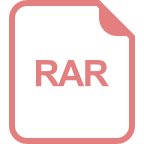
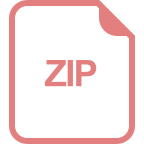
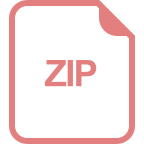
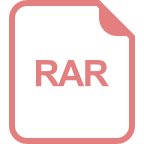
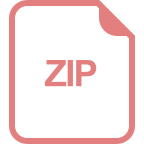
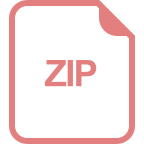
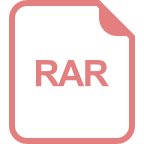
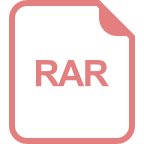
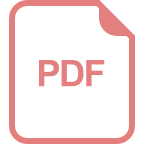
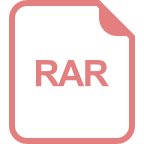
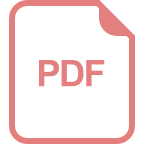