用python完成一个脚本,这个脚本从几个目录和这些目录的子目录下遍历所有的.h文件,在一个新的.cc文件中使用这些.h文件
时间: 2024-02-27 22:55:54 浏览: 21
好的,可以使用Python内置的os和shutil模块来完成这个任务。以下是一个示例代码,可以遍历指定目录下的.h文件,并将它们的路径写入一个新的.cc文件中:
```python
import os
import shutil
# 遍历的根目录
root_dir = "/path/to/root/dir"
# 遍历根目录及其子目录下的所有.h文件
header_files = []
for dirpath, dirs, files in os.walk(root_dir):
for file in files:
if file.endswith(".h"):
header_files.append(os.path.join(dirpath, file))
# 将所有.h文件的路径写入新的.cc文件
with open("output.cc", "w") as f:
for header_file in header_files:
f.write("#include \"%s\"\n" % header_file)
```
你可以将`root_dir`替换成你想要遍历的根目录,然后运行这个脚本即可。在`output.cc`文件中,会包含所有遍历到的.h文件的`#include`语句。
相关问题
用python完成一个脚本,从三个目录中和三个目录的子目录下遍历所有的.h文件,在一个新的.cc文件中使用这些.h文件
好的,我可以为您提供一些思路和代码实现。首先,我们需要使用Python的os库来遍历目录和文件,使用re库来匹配.h文件。然后,我们需要将匹配到的.h文件路径保存在一个列表中,并在一个新的.cc文件中使用这些路径。
以下是代码实现的示例:
```python
import os
import re
# 定义三个目录的路径
path1 = "path/to/directory1"
path2 = "path/to/directory2"
path3 = "path/to/directory3"
# 定义匹配.h文件的正则表达式
pattern = re.compile(r".*\.h$")
# 定义保存.h文件路径的列表
h_files = []
# 遍历三个目录及其子目录下的所有文件
for root, dirs, files in os.walk(path1):
for file in files:
if pattern.match(file):
h_files.append(os.path.join(root, file))
for root, dirs, files in os.walk(path2):
for file in files:
if pattern.match(file):
h_files.append(os.path.join(root, file))
for root, dirs, files in os.walk(path3):
for file in files:
if pattern.match(file):
h_files.append(os.path.join(root, file))
# 在新的.cc文件中使用.h文件路径
with open("new_file.cc", "w") as f:
for file_path in h_files:
f.write(f'#include "{file_path}"\n')
```
这段代码会遍历三个目录及其子目录下的所有文件,匹配出.h文件并将其路径保存在h_files列表中。最后,在新的.cc文件中使用这些.h文件路径,以#include的形式引入。您可以根据实际情况修改目录路径和文件名。
Python生成一个获取在不同目录下所有.h文件的脚本。并在.cc文件中使用这个些.h文件
可以使用Python的os和pathlib库来获取不同目录下所有的 `.h` 文件,并在 `.cc` 文件中使用这些 `.h` 文件。以下是一个示例代码:
```python
import os
import pathlib
# 设置目录路径
dir_paths = ['/path/to/dir1', '/path/to/dir2']
# 获取所有的 .h 文件路径
h_files = []
for dir_path in dir_paths:
for root, dirs, files in os.walk(dir_path):
h_files += [os.path.join(root, f) for f in files if f.endswith('.h')]
# 生成 all.h 文件
with open('all.h', 'w') as all_h_file:
for h_file in h_files:
# 写入 #include 指令
all_h_file.write(f'#include "{pathlib.Path(h_file).relative_to(os.getcwd())}"\n')
# 生成 all.cc 文件
with open('all.cc', 'w') as all_cc_file:
for h_file in h_files:
# 写入 #include 指令
all_cc_file.write(f'#include "{pathlib.Path(h_file).relative_to(os.getcwd())}"\n')
# 写入其他代码
all_cc_file.write('int main() {\n')
all_cc_file.write(' // TODO: Add your code here\n')
all_cc_file.write(' return 0;\n')
all_cc_file.write('}\n')
```
这个代码会在当前目录下生成一个 `all.h` 文件和一个 `all.cc` 文件,分别包含了所有的 `.h` 文件和一些示例代码。需要注意的是,这个代码假设所有的 `.h` 文件都以 `.h` 为后缀,如果文件后缀不同,需要根据实际情况进行调整。同时,这个代码会遍历所有子目录,如果不需要遍历子目录,需要对 `os.walk()` 函数进行调整。
相关推荐
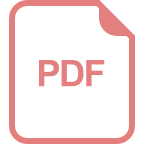












