请使用C语言帮我写一个程序,在linux系统上调用usb摄像头,获取帧图像数据后,调用另一个图像识别与处理程序app,处理完成后返回一个标识符,根据标识符通过串口有发给下位机一个标识符
时间: 2024-05-13 19:19:30 浏览: 248
以下是一个简单的代码示例,实现了调用USB摄像头获取帧图像数据并将其传递给图像识别程序进行处理,最后将处理结果发送给串口:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <errno.h>
#include <sys/ioctl.h>
#include <sys/mman.h>
#include <linux/videodev2.h>
#define CAMERA_DEVICE "/dev/video0" // 摄像头设备
#define IMAGE_WIDTH 640 // 图像宽度
#define IMAGE_HEIGHT 480 // 图像高度
#define IMAGE_SIZE (IMAGE_WIDTH * IMAGE_HEIGHT * 3) // 图像大小
#define APP_PATH "./app" // 图像识别程序路径
#define SERIAL_DEVICE "/dev/ttyS0" // 串口设备
#define RESULT_FLAG "OK" // 处理结果标识符
int main()
{
// 打开摄像头设备
int fd_camera = open(CAMERA_DEVICE, O_RDWR);
if (fd_camera == -1)
{
perror("Failed to open camera");
return -1;
}
// 设置摄像头采集参数
struct v4l2_capability cap;
struct v4l2_format fmt;
struct v4l2_requestbuffers req;
struct v4l2_buffer buf;
void* buffer_start;
memset(&cap, 0, sizeof(cap));
memset(&fmt, 0, sizeof(fmt));
memset(&req, 0, sizeof(req));
memset(&buf, 0, sizeof(buf));
cap.capabilities = V4L2_CAP_VIDEO_CAPTURE;
if (ioctl(fd_camera, VIDIOC_QUERYCAP, &cap) == -1)
{
perror("Failed to query camera capabilities");
return -1;
}
fmt.type = V4L2_BUF_TYPE_VIDEO_CAPTURE;
fmt.fmt.pix.width = IMAGE_WIDTH;
fmt.fmt.pix.height = IMAGE_HEIGHT;
fmt.fmt.pix.pixelformat = V4L2_PIX_FMT_RGB24;
fmt.fmt.pix.field = V4L2_FIELD_INTERLACED;
if (ioctl(fd_camera, VIDIOC_S_FMT, &fmt) == -1)
{
perror("Failed to set camera format");
return -1;
}
req.count = 1;
req.type = V4L2_BUF_TYPE_VIDEO_CAPTURE;
req.memory = V4L2_MEMORY_MMAP;
if (ioctl(fd_camera, VIDIOC_REQBUFS, &req) == -1)
{
perror("Failed to request camera buffers");
return -1;
}
memset(&buf, 0, sizeof(buf));
buf.type = V4L2_BUF_TYPE_VIDEO_CAPTURE;
buf.memory = V4L2_MEMORY_MMAP;
buf.index = 0;
if (ioctl(fd_camera, VIDIOC_QUERYBUF, &buf) == -1)
{
perror("Failed to query camera buffer");
return -1;
}
buffer_start = mmap(NULL, buf.length, PROT_READ | PROT_WRITE, MAP_SHARED, fd_camera, buf.m.offset);
if (buffer_start == MAP_FAILED)
{
perror("Failed to map camera buffer");
return -1;
}
if (ioctl(fd_camera, VIDIOC_STREAMON, &buf.type) == -1)
{
perror("Failed to start camera stream");
return -1;
}
// 打开串口设备
int fd_serial = open(SERIAL_DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd_serial == -1)
{
perror("Failed to open serial");
return -1;
}
// 循环获取图像数据并进行处理
while (1)
{
// 获取一帧图像
if (ioctl(fd_camera, VIDIOC_QBUF, &buf) == -1)
{
perror("Failed to queue camera buffer");
return -1;
}
if (ioctl(fd_camera, VIDIOC_DQBUF, &buf) == -1)
{
perror("Failed to dequeue camera buffer");
return -1;
}
// 调用图像识别程序进行处理
char cmd[1024];
sprintf(cmd, "%s %d %d", APP_PATH, IMAGE_WIDTH, IMAGE_HEIGHT);
FILE* pipe = popen(cmd, "w");
if (!pipe)
{
perror("Failed to open pipe");
return -1;
}
fwrite(buffer_start, buf.bytesused, 1, pipe);
pclose(pipe);
// 发送处理结果给下位机
write(fd_serial, RESULT_FLAG, strlen(RESULT_FLAG));
}
// 关闭摄像头设备和串口设备
if (ioctl(fd_camera, VIDIOC_STREAMOFF, &buf.type) == -1)
{
perror("Failed to stop camera stream");
return -1;
}
munmap(buffer_start, buf.length);
close(fd_camera);
close(fd_serial);
return 0;
}
```
以上代码仅供参考,实际使用时需要根据实际情况进行修改。
阅读全文
相关推荐
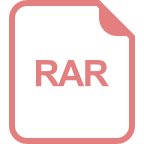
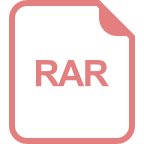
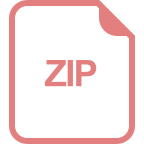
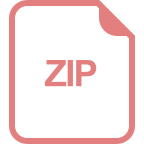
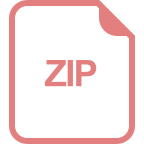
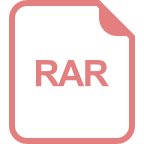
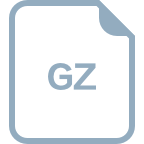
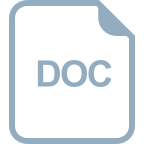
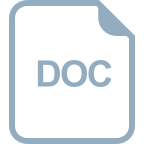
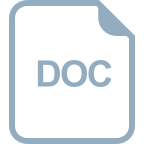
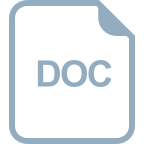
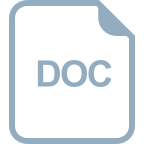
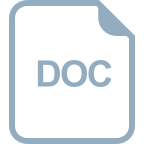
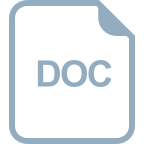
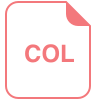
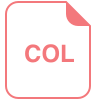
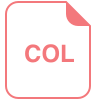