Verilog 语法
时间: 2023-08-03 08:18:56 浏览: 75
Verilog是一种硬件描述语言(HDL),用于描述数字电路和系统。以下是Verilog常用的语法元素:
1. 模块声明
Verilog程序由一个个模块(module)组成。模块声明包括模块名、输入和输出端口等。
```verilog
module module_name (input port1, input port2, output port3);
// 模块内部代码
endmodule
```
2. 数据类型
Verilog支持多种数据类型,包括位、字、整数、浮点数等。
```verilog
// 位类型
reg [7:0] data; // 8位寄存器
// 字类型
reg [15:0] addr; // 16位寄存器
// 整数类型
integer count = 0;
// 浮点数类型
real value = 3.14;
```
3. 运算符
Verilog支持多种运算符,包括算术运算符、位运算符、逻辑运算符等。
```verilog
// 算术运算符
a + b; // 加
a - b; // 减
a * b; // 乘
a / b; // 除
a % b; // 取余
// 位运算符
a & b; // 按位与
a | b; // 按位或
a ^ b; // 按位异或
~a; // 按位取反
// 逻辑运算符
a && b; // 逻辑与
a || b; // 逻辑或
!a; // 逻辑取反
```
4. 控制语句
Verilog支持多种控制语句,包括条件语句、循环语句和跳转语句等。
```verilog
// 条件语句
if (condition) begin
// 代码块
end
else begin
// 代码块
end
// 循环语句
for (i = 0; i < 10; i = i + 1) begin
// 代码块
end
// 跳转语句
case (signal)
2'b00: // 代码块
2'b01: // 代码块
2'b10: // 代码块
2'b11: // 代码块
endcase
```
5. 实例化模块
Verilog允许在一个模块中实例化另一个模块。
```verilog
module module1 (input port1, output port2);
// 模块内部代码
endmodule
module module2 (input port3, output port4);
module1 m1 (.port1(port3), .port2(port4));
endmodule
```
以上是Verilog常用的语法元素,掌握这些基本语法是学习和设计FPGA电路的基础。
相关推荐
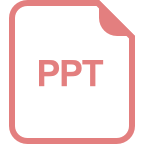














