编写一个Java程序,使用LocalDate、LocalTime、LocalDateTime、ZoneId、ZonedDateTime和Instant类完成以下操作:
时间: 2023-11-09 19:51:22 浏览: 121
使用Java的日期和时间API完成以下操作:
1. 创建一个LocalDate对象,表示当前日期。
2. 创建一个LocalTime对象,表示当前时间。
3. 创建一个LocalDateTime对象,表示当前日期和时间。
4. 使用ZoneId.of()方法创建一个ZoneId对象,表示指定的时区。
5. 使用ZonedDateTime类的now()方法创建一个ZonedDateTime对象,表示当前日期和时间以及指定的时区。
6. 使用Instant类的now()方法创建一个表示当前时间戳的Instant对象。
相关问题
LocalDate LocalDateTime
LocalDate和LocalDateTime是Java 8中的日期时间类。LocalDate表示日期,不包含时间信息,而LocalDateTime表示日期和时间。
引用[1]中的代码演示了如何从Instant对象和ZoneId对象创建LocalDateTime、LocalDate和LocalTime对象。通过ofInstant方法,我们可以将一个Instant对象转换为LocalDateTime对象,并使用toLocalDate和toLocalTime方法分别获取日期和时间。
引用[2]中的代码是一个工具方法,用于将Date对象转换为LocalDateTime对象。它使用了Date对象的toInstant方法将其转换为Instant对象,然后使用atZone方法将其转换为带有系统默认时区的ZonedDateTime对象,最后使用toLocalDateTime方法将其转换为LocalDateTime对象。
引用[3]中的代码展示了创建LocalDate对象的两种方法。第一种方法是使用now方法获取当前日期,第二种方法是使用of方法通过指定的年、月、日来设置日期。
所以,LocalDate用于表示日期,LocalDateTime用于表示日期和时间。您可以根据需要选择使用哪个类来处理日期和时间的操作。
Java8 时间戳转LocalTime LocalDate
以下是Java8中时间戳转换为LocalTime和LocalDate的示例代码[^1]:
1. 时间戳转LocalTime:
```java
import java.time.Instant;
import java.time.LocalTime;
import java.time.ZoneId;
public class TimestampToLocalTimeExample {
public static void main(String[] args) {
long timestamp = System.currentTimeMillis();
Instant instant = Instant.ofEpochMilli(timestamp);
LocalTime localTime = instant.atZone(ZoneId.systemDefault()).toLocalTime();
System.out.println("LocalTime: " + localTime);
}
}
```
2. 时间戳转LocalDate:
```java
import java.time.Instant;
import java.time.LocalDate;
import java.time.ZoneId;
public class TimestampToLocalDateExample {
public static void main(String[] args) {
long timestamp = System.currentTimeMillis();
Instant instant = Instant.ofEpochMilli(timestamp);
LocalDate localDate = instant.atZone(ZoneId.systemDefault()).toLocalDate();
System.out.println("LocalDate: " + localDate);
}
}
```
这些示例代码演示了如何将时间戳转换为LocalTime和LocalDate对象。首先,我们使用Instant类将时间戳转换为Instant对象。然后,使用atZone方法将Instant对象转换为特定时区的ZonedDateTime对象。最后,使用toLocalTime方法将ZonedDateTime对象转换为LocalTime对象,或使用toLocalDate方法将ZonedDateTime对象转换为LocalDate对象。
阅读全文
相关推荐
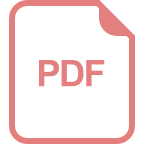
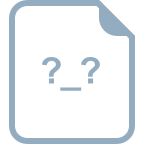
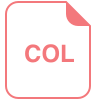
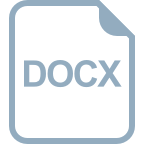
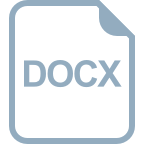
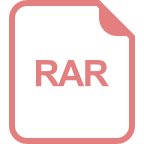
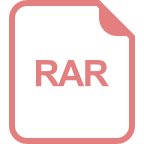
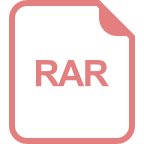
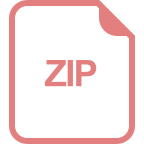
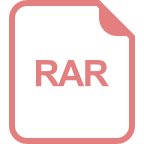
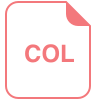
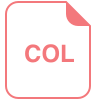



