本关任务:实现 mainstring.h 文件中的countCharNum函数,输出字符串中不同字符的个数,字符输出顺序按照字母序排列。
时间: 2024-12-24 20:20:46 浏览: 4
`mainstring.h` 文件中的 `countCharNum` 函数通常用于统计并返回给定字符串中所有唯一字符的数量,并按字母顺序显示。这个函数的基本步骤包括:
1. 定义一个名为 `countCharNum` 的函数,接受一个字符串参数。
2. 使用标准库中的容器(如 `std::unordered_map` 或 `std::map`),存储每个字符及其出现次数。
3. 遍历输入字符串,对于每一个字符,检查它是否已经在 map 中,如果已存在,则增加计数,否则添加新键值对(字符,1)。
4. 对 map 进行排序,使得字符按照字母顺序输出。可以使用 `std::sort` 和自定义比较函数(如 `[](const pair<char, int>& p) { return p.first; }`)。
5. 输出每个字符以及对应的出现次数。
以下是该函数的一个简单实现示例(假设使用 C++ 并且已经包含了 `<iostream>` 和 `<string>`):
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
#include <algorithm>
// 自定义字符比较函数
struct CompareChars {
bool operator()(char a, char b) { return a < b; }
};
void countCharNum(const std::string& inputStr) {
std::unordered_map<char, int> charCount;
for (char c : inputStr) {
if (isalpha(c)) { // 只处理字母,忽略非字母字符
charCount[c]++;
}
}
std::sort(charCount.begin(), charCount.end(), CompareChars());
for (const auto &pair : charCount) {
std::cout << pair.first << ": " << pair.second << "\n";
}
}
int main() {
std::string str = "Hello, World!";
countCharNum(str);
return 0;
}
```
阅读全文
相关推荐
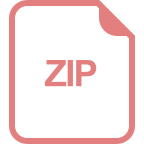
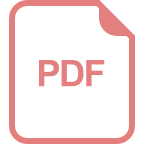
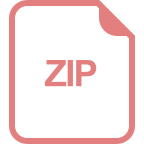
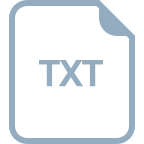
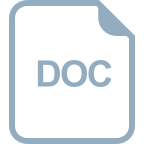
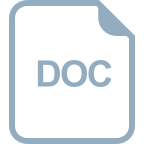
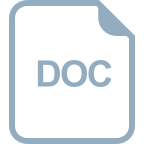
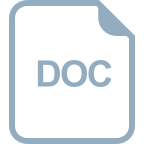
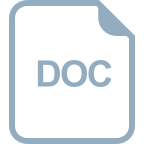









