怎样处理发送POST请求并携带自定义 headers?
时间: 2024-09-21 08:03:59 浏览: 40
在Java中,无论是使用`HttpURLConnection`还是`Apache HttpClient`,发送POST请求并携带自定义headers都很简单,下面是一个例子:
### 使用`HttpURLConnection`:
```java
URL url = new URL("http://example.com/api");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置POST请求方法
connection.setRequestMethod("POST");
// 添加自定义header
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Authorization", "Bearer your_token");
// 创建请求体,这里以JSON为例
String requestBody = "{\"key\":\"value\"}";
// 写入请求体
OutputStream outputStream = connection.getOutputStream();
outputStream.write(requestBody.getBytes(StandardCharsets.UTF_8));
outputStream.flush();
outputStream.close();
// 获取响应
try (BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()))) {
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
System.out.println(response.toString());
}
// 关闭连接
connection.disconnect();
```
### 使用`Apache HttpClient`:
```java
CloseableHttpResponse response;
HttpPost postRequest = new HttpPost("http://example.com/api");
postRequest.setHeader("Content-Type", "application/json");
postRequest.setHeader("Authorization", "Bearer your_token");
try {
BasicHttpParams params = new BasicHttpParams();
HttpClientBuilder clientBuilder = HttpClientBuilder.create(params)
.setDefaultHeaders(Collections.singletonMap("Content-Type", "application/json"));
HttpClient httpClient = clientBuilder.build();
response = httpClient.execute(postRequest, new StringEntity(requestBody));
// ...处理响应...
} finally {
// 关闭连接
response.close();
httpClient.getConnectionManager().shutdown();
}
```
在上述示例中,你需要将"your_token"替换为你实际的访问令牌,并确保`requestBody`是你想要发送的数据(这里是JSON格式)。
阅读全文
相关推荐
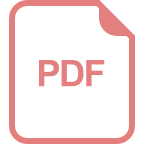
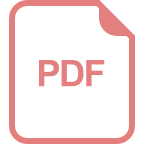
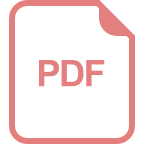
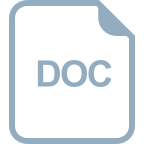
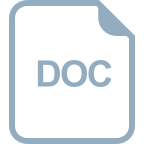






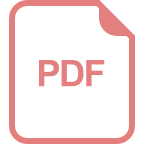
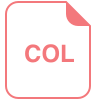





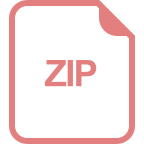