基于1.8.1pcl库改写以点p为起点的欧式聚类的c++代码
时间: 2024-04-11 17:27:57 浏览: 111
当然可以!下面是一个基于1.8.1版本的PCL库,用于以点p为起点的欧式聚类的C++代码示例:
```cpp
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/features/normal_3d.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/kdtree/kdtree.h>
#include <pcl/segmentation/extract_clusters.h>
int main()
{
// 创建点云对象
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
// 填充点云数据,此处省略
// 创建法线估计对象
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud(cloud);
// 创建kdtree对象,用于搜索邻域点
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>);
ne.setSearchMethod(tree);
// 估计法线
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals(new pcl::PointCloud<pcl::Normal>);
ne.setRadiusSearch(0.03); // 设置搜索半径
ne.compute(*cloud_normals);
// 创建欧式聚类对象
pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec;
ec.setInputCloud(cloud);
ec.setClusterTolerance(0.02); // 设置聚类的容差
ec.setMinClusterSize(100); // 设置每个聚类的最小大小
ec.setMaxClusterSize(25000); // 设置每个聚类的最大大小
ec.setSearchMethod(tree);
// 提取聚类结果
std::vector<pcl::PointIndices> cluster_indices;
ec.extract(cluster_indices);
// 输出聚类结果
int j = 0;
for (std::vector<pcl::PointIndices>::const_iterator it = cluster_indices.begin(); it != cluster_indices.end(); ++it)
{
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_cluster(new pcl::PointCloud<pcl::PointXYZ>);
for (std::vector<int>::const_iterator pit = it->indices.begin(); pit != it->indices.end(); ++pit)
cloud_cluster->points.push_back(cloud->points[*pit]);
cloud_cluster->width = cloud_cluster->points.size();
cloud_cluster->height = 1;
cloud_cluster->is_dense = true;
std::cout << "Cluster " << j << " has " << cloud_cluster->points.size() << " points." << std::endl;
j++;
}
return 0;
}
```
请注意,上述代码中的点云数据填充部分被省略了,你需要根据你的实际数据进行填充。此外,代码中的聚类参数也可以根据你的需求进行调整。
阅读全文
相关推荐
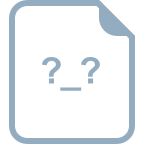
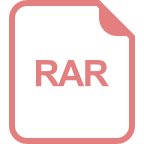
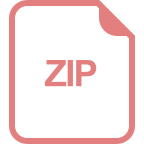
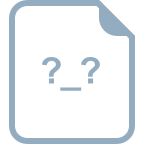
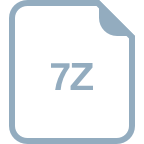
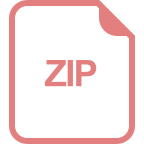
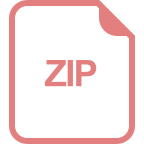
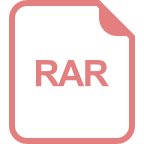
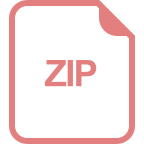
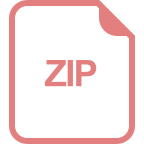
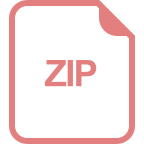
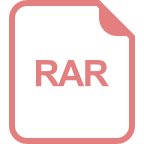