dma,freertos,zynq
时间: 2024-01-29 09:00:52 浏览: 22
DMA(Direct Memory Access,直接存储器访问)是一种计算机系统中的数据传输方式,其主要目的是在不需通过CPU的干预下,将数据在外设和内存之间进行高速传输。
FreeRTOS是一款开源的实时操作系统(RTOS),它专注于提供可靠性、高效性和可扩展性,用于嵌入式系统的开发。FreeRTOS可以帮助开发人员管理任务和资源,提供多任务并发操作的能力,并且具有广泛的硬件支持。
Zynq是赛灵思(Xilinx)公司开发的一系列基于ARM处理器和FPGA(Field-Programmable Gate Array,现场可编程门阵列)的可扩展平台。Zynq将高性能的ARM Cortex-A核心与灵活的可编程逻辑电路相结合,使得嵌入式系统的设计能够更加灵活、高效,并且可以满足不同应用的需求。
在使用Zynq进行嵌入式系统开发时,可以结合使用DMA和FreeRTOS来提高系统的效率和性能。DMA可以通过高速的数据传输减少CPU的负担,并且与FreeRTOS的任务管理机制相结合,可以实现更好的并发操作和资源管理。同时,Zynq平台提供了丰富的外设接口和可编程逻辑资源,使开发人员可以根据具体需求进行灵活的硬件设计和定制化开发。
总之,DMA、FreeRTOS和Zynq是在嵌入式系统开发中常用的技术和平台,它们可以相互结合,提供高效、可靠的解决方案,满足不同应用场景的需求。
相关问题
zynq freertos
Zynq是一种由Xilinx开发的可程式逻辑与嵌入式处理器相结合的片上系统(SoC)。它将可程式逻辑(FPGA)与处理器(Arm Cortex-A系列)集成在一起,提供了高度的灵活性和性能。
而FreeRTOS是一种开源的实时操作系统(RTOS),它专注于嵌入式系统的实时任务调度和资源管理。FreeRTOS具有轻量级、可裁剪、易于移植等特点,非常适合在资源受限的嵌入式系统中进行实时任务的管理。
Zynq与FreeRTOS结合使用可以充分发挥它们各自的优势。首先,由于Zynq具有可程式逻辑,我们可以将一些外设或任务逻辑实现为硬件逻辑,以提高性能和效率。其次,Zynq的处理器可以运行FreeRTOS,充当任务调度器和资源管理器,通过实时调度算法和优先级级别,确保任务按时完成。
在使用Zynq和FreeRTOS的嵌入式系统中,我们可以将系统划分为多个实时任务,并为每个任务分配不同的优先级。根据任务的实时性要求,我们可以使用FreeRTOS提供的丰富的任务调度功能,包括互斥量、信号量、消息队列等,以实现任务间的通信和资源共享。
总的来说,Zynq和FreeRTOS的结合可以提供灵活、高性能、实时的嵌入式系统解决方案。通过充分利用Zynq的可程式逻辑和FreeRTOS的实时任务调度能力,我们可以构建出高度可靠、强实时性的嵌入式应用,并满足各种不同应用场景的要求。
zynq freertos smp
Zynq是一款由Xilinx开发的可扩展处理器系统。它结合了ARM处理器和可编程逻辑的优势,提供了强大的计算和灵活性。FreeRTOS是一个开源的实时操作系统,适用于嵌入式系统。SMP是Symmetric Multiprocessing的缩写,指的是多处理器系统中的对称多处理模式,其中所有处理器都是对等的,可以同时执行任务。
在Zynq平台上,FreeRTOS SMP可以实现多个处理器同时执行任务,提高系统的并发性能。每个处理器都有自己独立的寄存器状态保存寄存器(SPSR),并且IRQ模式可以用于提供给用户的中断处理。在FIQ模式下,R8~R12寄存器也有自己的命名,不需要额外的压栈和出栈操作。
因此,Zynq上的FreeRTOS SMP可以通过使用IRQ模式和独立的SPSR来支持多处理器系统,并实现对称多处理模式的任务调度和并发执行。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [freeRTOS移植——ZYNQ7000简介](https://blog.csdn.net/weixin_42385536/article/details/80639790)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
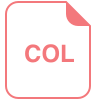











