tensorflow构建1维卷积
时间: 2023-09-02 14:02:06 浏览: 46
TensorFlow中的1维卷积是使用`tf.nn.conv1d`函数来实现的。该函数接受输入张量、卷积核权重和步幅等参数,并返回卷积后的结果。
首先,我们需要创建输入张量。在1维卷积中,输入张量的维度应为[batch_size, sequence_length, input_channels],其中batch_size是每批次的样本数量,sequence_length是序列的长度,input_channels是每个位置上的特征数。
接下来,我们需要定义卷积核权重。卷积核是卷积运算的参数,通过对输入张量的每个位置进行滑动窗口的计算得到输出特征。卷积核权重的维度应为[filter_width, input_channels, output_channels],其中filter_width是滑动窗口的宽度,input_channels是输入张量的特征数,output_channels是输出特征的数量。
然后,我们可以通过调用`tf.nn.conv1d`函数来进行卷积运算。该函数接受输入张量、卷积核权重、步幅和填充方式等参数,并返回卷积后的结果。步幅定义了滑动窗口的移动步长,填充方式可以选择是'SAME'(保持输出特征数量与输入特征数量相同)还是'VALID'(不填充)。
最后,我们可以运行计算图,并获得卷积后的结果。
下面是一个示例代码:
```python
import tensorflow as tf
# 创建输入张量
batch_size = 1
sequence_length = 10
input_channels = 1
input_tensor = tf.placeholder(tf.float32, shape=[batch_size, sequence_length, input_channels])
# 定义卷积核权重
filter_width = 3
output_channels = 1
filter_weights = tf.Variable(tf.random_normal([filter_width, input_channels, output_channels]))
# 进行卷积运算
strides = 1
padding = 'VALID'
conv_output = tf.nn.conv1d(input_tensor, filter_weights, stride=strides, padding=padding)
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
# 准备输入数据
input_data = [[[1.0], [2.0], [3.0], [4.0], [5.0], [6.0], [7.0], [8.0], [9.0], [10.0]]]
# 运行计算图
result = sess.run(conv_output, feed_dict={input_tensor: input_data})
print(result)
```
以上代码展示了如何使用TensorFlow构建一个简单的1维卷积。输入张量是一个10个元素的一维序列,卷积核是一个3x1的矩阵。将其运行后,可以获得卷积后的结果。
相关推荐
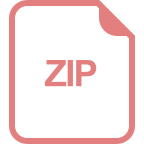














