C# 点击PDF 下载按钮,弹出选择文件存放位置,点击确定后下载PDF,文件名称后台默认
时间: 2024-09-06 11:02:17 浏览: 45
在C#中实现点击按钮下载PDF文件,并弹出对话框让用户选择文件存放位置,可以使用WebClient类或者HttpClient类来下载文件,并结合SaveFileDialog类来实现文件保存的对话框。以下是一个简单的示例代码:
1. 首先,需要添加对System.Net和System.Windows.Forms的引用,因为这两个命名空间中包含了实现这些功能所需的类。
```csharp
using System.Net;
using System.IO;
using System.Windows.Forms;
```
2. 在按钮点击事件中,使用SaveFileDialog类来弹出保存文件的对话框,用户可以在这里选择保存位置和文件名。
```csharp
private void downloadButton_Click(object sender, EventArgs e)
{
SaveFileDialog saveFileDialog = new SaveFileDialog();
saveFileDialog.Filter = "PDF文件|*.pdf";
saveFileDialog.Title = "保存PDF文件";
if (saveFileDialog.ShowDialog() == DialogResult.OK)
{
string fileName = saveFileDialog.FileName;
string fileExtension = Path.GetExtension(fileName);
if (fileExtension != ".pdf")
{
fileName += ".pdf";
}
// 使用WebClient类下载文件
using (WebClient webClient = new WebClient())
{
webClient.DownloadFileCompleted += (o, args) =>
{
MessageBox.Show("下载完成");
};
webClient.DownloadProgressChanged += (o, args) =>
{
// 这里可以更新进度条
};
webClient.DownloadFileAsync(new Uri("http://example.com/path/to/your.pdf"), fileName);
}
}
}
```
在这段代码中,首先创建了一个SaveFileDialog对象,并设置了文件过滤器,只允许用户选择PDF文件。然后,检查用户选择的文件名是否为PDF格式,如果不是,则自动添加.pdf扩展名。之后,使用WebClient的DownloadFileAsync方法异步下载文件,下载过程中可以提供进度条的更新,下载完成后会弹出一个消息框通知用户。
阅读全文
相关推荐
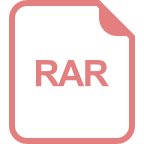
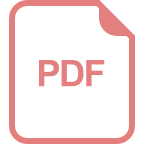
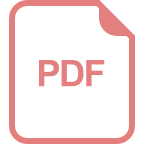
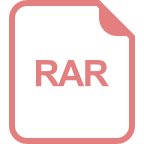
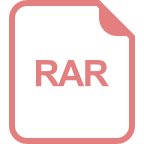
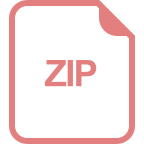
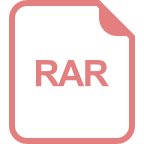
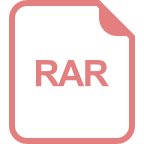
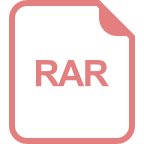
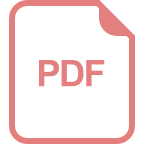
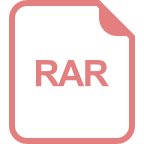
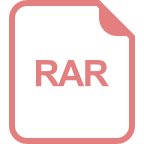
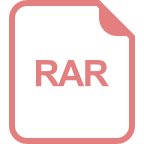
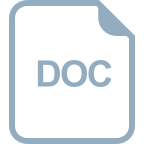
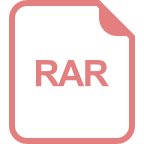
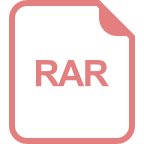
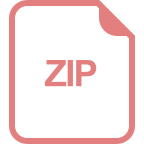