UnicodeDecodeError: 'utf-8' codec can't decode byte 0xab in position 78: illegal multibyte sequence
时间: 2023-11-09 09:53:26 浏览: 41
这个错误是UnicodeDecodeError: 'utf-8' codec can't decode byte 0xab in position 78: illegal multibyte sequence。这个错误的原因是在使用utf-8解码时遇到了非法的多字节序列。解决这个问题的方法是在open()函数中添加encoding='utf-8'参数,确保以utf-8编码打开文件。例如,可以使用以下代码来解决这个问题:
with open('file.txt', 'r', encoding='utf-8') as f:
# code here
相关问题
UnicodeDecodeError: 'gbk' codec can't decode byte 0xab in position 2049: illegal multibyte sequence
当出现UnicodeDecodeError: 'gbk' codec can't decode byte 0xab in position 2049: illegal multibyte sequence错误时,这意味着在使用'gbk'编解码器解码时,遇到了非法的多字节序列。这通常是由于文件的编码与所使用的编解码器不匹配导致的。
解决这个问题的方法是使用正确的编解码器来读取文件。首先,你需要确定文件的实际编码格式。可以尝试使用其他编解码器,如'utf-8'或'latin-1'来读取文件。如果仍然出现错误,可能需要使用更高级的编解码器库,如chardet来自动检测文件的编码格式。
以下是一个示例代码,演示如何使用不同的编解码器来读取文件:
```python
# 使用'utf-8'编解码器读取文件
with open('file.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
# 使用'latin-1'编解码器读取文件
with open('file.txt', 'r', encoding='latin-1') as f:
content = f.read()
print(content)
```
请注意,你需要将代码中的'file.txt'替换为你实际的文件路径。
UnicodeDecodeError: 'gbk' codec can't decode byte 0xab in position 360: illegal multibyte sequence
UnicodeDecodeError是一个编码解码错误,通常发生在尝试将字节序列解码为字符串时。这个错误通常是由于编码不匹配或包含无效的字节序列导致的。在你提供的引用中,报错信息是"UnicodeDecodeError: 'gbk' codec can't decode byte 0xab in position 360: illegal multibyte sequence"。这个错误表示在使用'gbk'编解码器将字节序列解码为字符串时,遇到了无效的多字节序列。
解决这个错误的方法有几种,具体取决于你的使用场景和数据来源。以下是一些可能的解决方法:
1. 使用正确的编码方式进行解码:根据报错信息,你可以尝试使用正确的编码方式进行解码。在这种情况下,你可以尝试使用'utf-8'编码方式进行解码,因为'utf-8'是一种常用的编码方式,支持更广泛的字符集。你可以使用以下代码进行尝试:
```python
data.decode('utf-8')
```
2. 指定正确的编码方式:如果你知道数据的正确编码方式,你可以直接指定该编码方式进行解码。例如,如果你知道数据是使用'gbk'编码的,你可以使用以下代码进行解码:
```python
data.decode('gbk')
```
3. 处理无效的字节序列:如果你的数据中包含无效的字节序列,你可以尝试使用错误处理机制来处理它们。例如,你可以使用'ignore'参数来忽略无效的字节序列,或者使用'replace'参数来替换无效的字节序列。以下是使用'ignore'参数的示例代码:
```python
data.decode('gbk', errors='ignore')
```
请注意,具体的解决方法取决于你的使用场景和数据来源。你需要根据实际情况选择适合的解决方法。
相关推荐
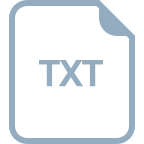
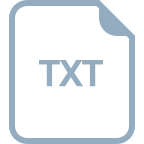
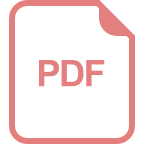












