QT使用tcp链接例子
时间: 2024-10-22 11:23:35 浏览: 16
在QT中,使用TCP连接可以通过`QTcpSocket`类来实现。以下是一个基本的例子,展示了如何创建一个客户端和服务端的TCP连接:
**服务端(Server.cpp):**
```cpp
#include <QTcpServer>
#include <QObject>
class Server : public QObject {
Q_OBJECT
public:
explicit Server(QObject *parent = nullptr) : QObject(parent) {
server = new QTcpServer(this);
connect(server, &QTcpServer::newConnection, this, &Server::handleNewConnection);
if (!server->listen(QHostAddress::Any, 12345)) { // 使用任意IP地址监听12345端口
qCritical() << "Listen failed: " << server->errorString();
}
}
private slots:
void handleNewConnection() {
QTcpSocket *client = server->nextPendingConnection();
connect(client, &QTcpSocket::readyRead, this, &Server::readData);
connect(client, &QTcpSocket::disconnected, this, &Server::connectionClosed);
}
private:
QTcpServer *server;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Server server;
return app.exec();
}
```
**客户端(Client.cpp):**
```cpp
#include <QTcpSocket>
#include <QObject>
#include <QApplication>
class Client : public QObject {
Q_OBJECT
public:
explicit Client(const QString& host, quint16 port, QObject *parent = nullptr)
: QObject(parent), socket(new QTcpSocket(this))
{
connect(socket, &QTcpSocket::connected, this, &Client::onConnected);
connect(socket, &QTcpSocket::disconnected, this, &Client::onDisconnected);
connect(socket, &QTcpSocket::readyRead, this, &Client::readData);
socket->connectToHost(host, port); // 连接到服务器
}
private slots:
void onConnected() {
socket->write("Hello from client!"); // 发送数据到服务器
}
void readData() {
QByteArray data = socket->readAll();
qDebug() << "Received data: " << data;
}
void onDisconnected() {
qDebug() << "Connection closed";
}
private:
QTcpSocket *socket;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Client client("localhost", 12345); // 本地主机,假设服务端在本机运行
return app.exec();
}
```
在这个例子中,服务端创建一个`QTcpServer`并监听,当有新的连接请求时,它会创建一个新的`QTcpSocket`实例并传递给`handleNewConnection`槽函数。客户端则连接到服务端,并在连接成功后发送消息。
阅读全文
相关推荐





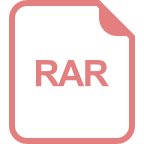












