vue使用ffmpeg,demo
时间: 2024-07-05 13:00:44 浏览: 168
在Vue.js中使用FFmpeg进行视频处理通常涉及到前端调用后端提供的API或者使用一些Node.js库(如`fluent-ffmpeg`)来处理视频操作,因为直接在浏览器中运行FFmpeg是受限的,出于安全和性能考虑。以下是一个简单的步骤和一个基础的示例:
1. 安装依赖:首先在项目中安装Node.js,然后安装处理视频的库,比如`fluent-ffmpeg`。在项目目录下运行:
```bash
npm install fluent-ffmpeg --save
```
2. 创建后端API:在Node.js或类似的后端框架(如Express)中,创建一个API来接收视频文件和执行FFmpeg命令。例如,你可以创建一个POST请求接收视频文件,并执行转换操作:
```javascript
// server.js 或 backend/api/videoProcessor.js
const express = require('express');
const ffmpeg = require('fluent-ffmpeg');
const app = express();
app.use(express.json());
app.post('/convert', async (req, res) => {
try {
const videoFile = req.files.video;
const outputFilePath = `./temp/${videoFile.name}`;
// 使用FFmpeg转换视频
const command = ffmpeg(videoFile.path)
.setOutputOptions('-c:v libx264 -preset veryfast')
.save(outputFilePath);
await command.on('end', () => {
res.status(200).json({ message: 'Video processed successfully', resultPath: outputFilePath });
})
.on('error', (err) => {
console.error(err);
res.status(500).json({ error: 'Error processing video' });
});
} catch (error) {
res.status(500).json({ error: 'Internal server error' });
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
3. Vue组件调用API:在Vue组件中,你需要使用axios或其他HTTP库来发送POST请求到后端:
```html
<!-- video-processing.vue -->
<template>
<div>
<input type="file" @change="uploadVideo" />
<button @click="convertVideo">Convert Video</button>
<div v-if="convertedVideo">
<video :src="convertedVideoUrl" controls></video>
</div>
<div v-if="error">{{ error }}</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
convertedVideo: false,
convertedVideoUrl: '',
error: ''
};
},
methods: {
uploadVideo(e) {
this.file = e.target.files;
},
convertVideo() {
if (!this.file) return;
this.$axios.post('/api/convert', { video: this.file }).then(response => {
this.convertedVideo = true;
this.convertedVideoUrl = response.data.resultPath;
}).catch(error => {
this.error = error.message;
});
}
}
};
</script>
```
阅读全文
相关推荐
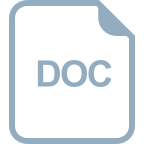
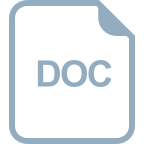
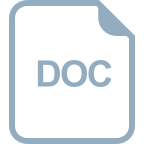
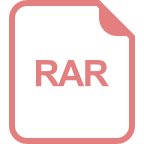
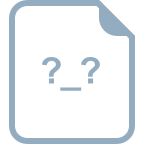
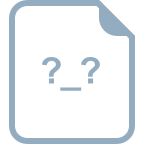
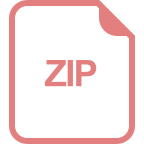
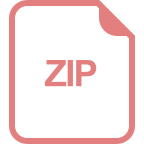
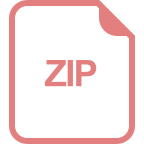
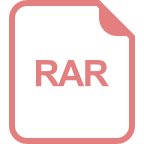
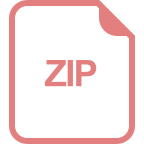
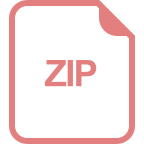
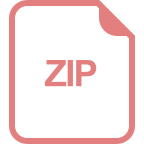
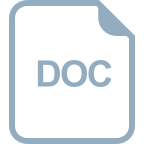
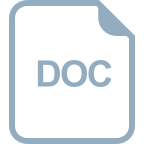
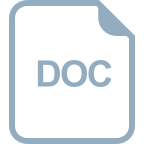
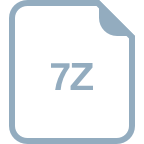
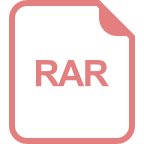
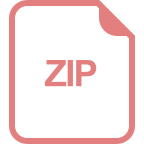