Python使用apply函数对列表做处理
时间: 2024-11-29 08:36:20 浏览: 5
Python中的`apply()`函数可以用来将一个函数应用于一个序列(如列表)的每个元素上。这是通过传入一个函数和一个可迭代对象(如列表)作为参数实现的。这里有一个简单的例子:
```python
def my_function(element):
return element * 2
# 使用apply对列表进行处理
alist = [1, 2, 3, 4, 5]
applied_list = map(my_function, alist)
# 或者使用内置的列表推导式,因为map在Python 3中已过时
applied_list = [my_function(i) for i in alist]
print(applied_list) # 输出:[2, 4, 6, 8, 10]
```
在这个例子中,`my_function`会被应用到`alist`的每一个元素上,返回的新列表包含了原列表中每个元素翻倍的结果。
请注意,尽管上述示例展示了`map()`函数的用法,但现代的Python版本更推荐使用列表推导式或其他内建函数,如`list comprehension`代替`apply()`,因为它已经从Python 3开始被弃用。
相关问题
实例 Python使用apply函数对列表做处理
Python的`apply()`函数并非内置函数,但在早期版本(如pandas库的pre-0.23)中确实存在,用于对数组应用特定操作。然而,在现代的pandas库(0.23及以上版本)中,已经改用了更推荐的方式,比如`map()`函数。
如果你想了解如何使用`apply()`函数(虽然现在不常用),它通常是在NumPy或者旧版pandas中用于迭代数组元素并应用某个函数:
```python
import numpy as np
# 假设我们有一个numpy数组
numbers = np.array([1, 2, 3, 4, 5])
# 使用apply()(注意这不是pandas的apply,这里指的是NumPy)
def square(x):
return x**2
squared_numbers = np.apply_along_axis(square, axis=0, arr=numbers)
print(squared_numbers) # 输出: array([1, 4, 9, 16, 25])
```
然而,现代的做法会建议使用`np.map()`或`pandas.Series.map()`,因为它们更直观易懂:
```python
# pandas示例
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
squared_df = df['A'].map(lambda x: x**2)
print(squared_df) # 输出: 0 1
# 1 4
# 2 9
# Name: A, dtype: int64
```
python dataframe apply函数
Python中的DataFrame apply函数是一种数据处理函数,它可以对DataFrame中的每一列或每一行进行函数操作。apply函数可以接受一个函数作为参数,这个函数可以是自定义的函数或者是Python内置的函数。apply函数的返回值是一个Series或DataFrame对象,它们的长度与原始DataFrame的长度相同。apply函数的使用可以大大简化数据处理的过程,提高数据处理的效率。
阅读全文
相关推荐
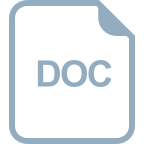
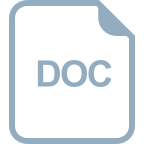
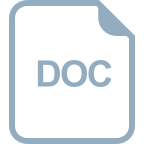
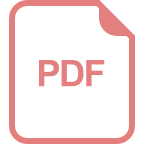
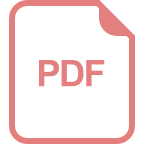






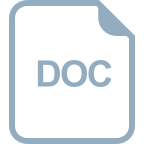
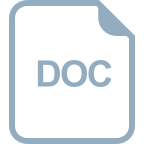