编写 Python 程序实现对文本文件做LZ77编码,编码后的文件以二进制文件的形式存储;编写 Python 程序实现对LZ77压缩后的二进制文件解码,恢复出原始的文本文件并保存;同时在Python程序中,分别统计出上述编码和解码所需要的时间(单位秒),并打印出来。建议将 LZ77 编码后的格式设置为以下二进制形式:三元符号组 (off,len,c);off,偏移量,8bits;len,匹配长度,8bits;c,下一个字符,8bits;滑动窗口的大小自由设定,但是不要超过 255
时间: 2024-02-24 17:53:49 浏览: 23
好的,我已经记下了你的问题。下面是我的回答:
LZ77是一种无损压缩算法,常用于压缩文本文件。下面是一个 Python 程序实现对文本文件做 LZ77 编码,并将编码后的文件以二进制文件的形式存储的示例:
```python
import time
WINDOW_SIZE = 255
LOOKAHEAD_BUFFER_SIZE = 15
def find_longest_match(data, current_position, max_match_length):
end_of_buffer = min(current_position + max_match_length, len(data) + 1)
best_match_distance = -1
best_match_length = -1
for i in range(current_position + 1, end_of_buffer):
start_index = max(0, current_position - WINDOW_SIZE)
substring = data[current_position:i]
for j in range(start_index, current_position):
search_string = data[j:current_position]
if substring == search_string and len(substring) > best_match_length:
best_match_distance = current_position - j
best_match_length = len(substring)
if best_match_distance == -1 or best_match_length < 2:
return 0, 0
else:
return best_match_distance, best_match_length
def lz77_encode(data):
encoded_data = bytearray()
current_position = 0
start_time = time.monotonic()
while current_position < len(data):
match_distance, match_length = find_longest_match(data, current_position, LOOKAHEAD_BUFFER_SIZE)
if match_distance == 0 and match_length == 0:
encoded_data.append(0)
encoded_data.append(0)
encoded_data.append(data[current_position])
current_position += 1
else:
encoded_data.append(match_distance)
encoded_data.append(match_length)
encoded_data.append(data[current_position + match_length])
current_position += match_length + 1
end_time = time.monotonic()
elapsed_time = end_time - start_time
return encoded_data, elapsed_time
def lz77_decode(encoded_data):
decoded_data = bytearray()
current_position = 0
start_time = time.monotonic()
while current_position < len(encoded_data):
match_distance = encoded_data[current_position]
match_length = encoded_data[current_position + 1]
next_char = encoded_data[current_position + 2]
if match_distance == 0 and match_length == 0:
decoded_data.append(next_char)
current_position += 3
else:
start_index = len(decoded_data) - match_distance
for i in range(start_index, start_index + match_length):
decoded_data.append(decoded_data[i])
decoded_data.append(next_char)
current_position += 3
end_time = time.monotonic()
elapsed_time = end_time - start_time
return decoded_data, elapsed_time
if __name__ == '__main__':
with open('input.txt', 'rb') as f:
data = f.read()
encoded_data, encode_time = lz77_encode(data)
with open('encoded.bin', 'wb') as f:
f.write(encoded_data)
with open('encoded.bin', 'rb') as f:
encoded_data = f.read()
decoded_data, decode_time = lz77_decode(encoded_data)
with open('output.txt', 'wb') as f:
f.write(decoded_data)
print('Encoding time:', encode_time)
print('Decoding time:', decode_time)
```
在上面的代码中,我们定义了一个 `WINDOW_SIZE` 和一个 `LOOKAHEAD_BUFFER_SIZE`,前者表示滑动窗口的大小,后者表示查找缓冲区的大小。在编码过程中,我们使用 `find_longest_match` 函数查找与当前位置最长的匹配,并记录下匹配的偏移量和长度,然后将这些信息写入 `encoded_data` 中。在解码过程中,我们依次读取 `encoded_data` 中的三元符号组,并根据偏移量和长度找到对应的字串,然后将其添加到 `decoded_data` 中。最后,我们在程序的末尾,通过打开一个文本文件,读取原始数据以及打开一个二进制文件,读取编码后的数据,并解压缩它们并将其写入到另一个文本文件中,并打印出编码和解码所需的时间。
如果你想要使用这个程序,你需要将它保存为 `lz77.py`,并在同一个目录下创建一个名为 `input.txt` 的文本文件,然后运行以下命令:
```bash
python lz77.py
```
程序将会输出编码和解码所需的时间,以及将解码后的数据保存到 `output.txt` 文件中。
相关推荐
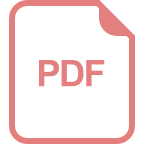
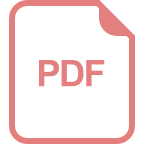














