new promise
时间: 2023-06-05 22:04:28 浏览: 85
Promise 是 JavaScript 中的一种用于处理异步操作的对象。它有两个主要的方法:`then` 和 `catch`。当你创建一个新的 Promise 对象时,你可以使用它来包装一个异步任务,并通过调用它的 `then` 方法来处理任务的结果,或者通过调用它的 `catch` 方法来处理任务中出现的错误。
例如:
```
const myPromise = new Promise((resolve, reject) => {
// 异步任务代码
if (/* 异步任务成功 */) {
resolve(/* 任务结果 */);
} else {
reject(/* 错误信息 */);
}
});
myPromise.then((result) => {
// 处理任务结果
}).catch((error) => {
// 处理错误信息
});
```
在这个例子中,我们创建了一个新的 Promise 对象,并在其中包装了一个异步任务。如果任务成功完成,我们调用 `resolve` 方法来传递任务结果;如果任务失败,我们调用 `reject` 方法来传递错误信息。然后我们使用 `then` 和 `catch` 方法来处理任务结果和错误信息。
相关问题
new Promise
The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value. It provides a way to handle asynchronous operations in a more readable and manageable way, using a set of methods such as `.then()`, `.catch()`, and `.finally()`.
A new Promise can be created using the `Promise` constructor, which takes a function called the executor function as its parameter. The executor function takes two arguments: `resolve` and `reject`. `resolve` is a function that is used to fulfill the promise and return the result of the operation. `reject` is a function that is used to reject the promise and return the error that occurred during the operation.
Here is an example of creating a new Promise:
```
const myPromise = new Promise((resolve, reject) => {
// perform some asynchronous operation
// if the operation is successful, call the resolve function with the result
// if the operation fails, call the reject function with an error message
});
```
Once the Promise is created, you can use the `.then()` method to handle the successful completion of the operation, and the `.catch()` method to handle any errors that occurred during the operation.
```
myPromise.then((result) => {
// handle successful completion and use the result
}).catch((error) => {
// handle error and display error message
});
```
The `.finally()` method can also be used to perform some cleanup tasks, regardless of whether the operation was successful or not.
js new Promise
JavaScript中的Promise是一种用于处理异步操作的对象。它可以将异步操作封装成一个Promise实例,通过链式调用的方式来处理异步操作的结果。
使用Promise可以更优雅地处理回调地狱(callback hell)问题,使代码更易读、维护和扩展。
创建一个Promise对象可以使用`new Promise()`语法,它接受一个执行器函数作为参数。执行器函数有两个参数:`resolve`和`reject`,分别用于成功和失败时的处理。
下面是一个简单的示例:
```javascript
const promise = new Promise((resolve, reject) => {
// 异步操作
setTimeout(() => {
const data = 'Hello, Promise!';
// 模拟异步操作成功
resolve(data);
// 模拟异步操作失败
// reject(new Error('Something went wrong!'));
}, 2000);
});
promise.then((result) => {
console.log(result); // 输出: Hello, Promise!
}).catch((error) => {
console.error(error); // 输出: Error: Something went wrong!
});
```
在上面的示例中,我们创建了一个Promise对象,并在2秒后模拟异步操作成功,然后通过`resolve`方法将结果传递给`then`方法进行处理。如果异步操作失败,可以通过`reject`方法将错误传递给`catch`方法进行处理。
相关推荐
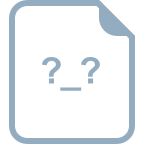












