生成一个调用第三方api代码
时间: 2023-07-15 19:15:39 浏览: 40
这里给出一个使用 Python 调用天气 API 的示例代码:
```python
import requests
# 请求 API
response = requests.get("http://api.openweathermap.org/data/2.5/weather?q=London&appid=your_app_id")
# 解析返回的 JSON 数据
data = response.json()
# 输出温度信息
print("温度为:", data["main"]["temp"])
```
需要注意的是,这里的 `your_app_id` 需要替换为自己在 openweathermap.org 上申请的 API Key。同时,请求的 URL 可以根据实际情况进行更改。
相关问题
用java生成一个调用第三方api代码
生成调用第三方API的代码需要以下步骤:
1. 确认第三方API的使用方式和协议,例如RESTful API、SOAP API、JSON API等。
2. 在Java中导入相关的API库或者使用HTTP请求库,例如HttpClient或者OkHttp。
3. 通过API提供的接口文档,构建相应的请求参数、请求头等信息。
4. 发送HTTP请求并获取API返回的响应数据。
5. 解析响应数据并处理异常情况。
下面是一个简单的使用Java调用第三方API的示例代码:
```
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class ApiCaller {
public static void main(String[] args) throws IOException {
// 待调用的API地址
String apiUrl = "https://api.example.com/some-endpoint";
// 构建HTTP连接
URL url = new URL(apiUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置HTTP请求头
connection.setRequestMethod("GET");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Authorization", "Bearer <your-access-token>");
// 发送HTTP请求
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// 读取API返回的响应数据
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 处理API返回的响应数据
System.out.println(response.toString());
} else {
// 处理API返回的错误信息
System.out.println("API调用失败,错误码为:" + responseCode);
}
connection.disconnect();
}
}
```
这是一个简单的GET请求示例,实际的调用方式会根据API的不同而有所差异。
java 调用第三方接口生成电子发票
以下是Java调用第三方接口生成电子发票的示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class InvoiceGenerator {
public static void main(String[] args) {
try {
// 设置请求参数
String url = "http://api.example.com/invoice";
String charset = "UTF-8";
String param1 = "value1";
String param2 = "value2";
String query = String.format("param1=%s¶m2=%s",
URLEncoder.encode(param1, charset),
URLEncoder.encode(param2, charset));
// 创建连接
URL api = new URL(url + "?" + query);
HttpURLConnection connection = (HttpURLConnection) api.openConnection();
connection.setRequestMethod("GET");
// 获取响应
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 处理响应
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上代码通过Java.net.HttpURLConnection类调用第三方接口生成电子发票。其中,设置请求参数时需要将参数进行编码,创建连接时使用URL类,获取响应时使用BufferedReader类,处理响应时将响应转换为字符串输出。
相关推荐
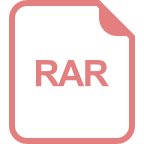
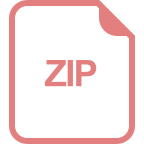












