用Python写一个Linux巡检脚本
时间: 2023-09-12 12:05:29 浏览: 163
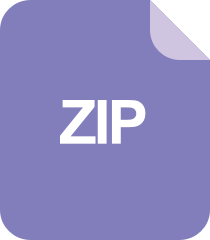
基于python的一个服务器巡检脚本+源代码+文档说明
### 回答1:
好的,下面是一个简单的Python脚本,可以用于巡检Linux系统的基本信息:
```
import platform
def check_system_info():
# 获取操作系统名称及版本
os_name = platform.system()
os_release = platform.release()
print("操作系统名称: ", os_name)
print("操作系统版本: ", os_release)
# 获取系统的主机名
host_name = platform.node()
print("主机名: ", host_name)
# 获取 CPU 信息
cpu_info = platform.processor()
print("CPU信息: ", cpu_info)
# 获取内存信息
mem_info = platform.platform()
print("内存信息: ", mem_info)
if __name__ == '__main__':
check_system_info()
```
这段代码可以帮助您收集系统的一些基本信息,如操作系统名称和版本、主机名、CPU信息和内存信息等。
### 回答2:
Python是一种非常强大和灵活的编程语言,可以用来编写各种类型的脚本。下面是一个简单的用Python编写的Linux巡检脚本:
```python
import subprocess
def check_disk_usage():
# 检查磁盘使用情况
cmd = "df -h"
output = subprocess.check_output(cmd, shell=True)
print("磁盘使用情况:")
print(output.decode())
def check_memory_usage():
# 检查内存使用情况
cmd = "free -m"
output = subprocess.check_output(cmd, shell=True)
print("内存使用情况:")
print(output.decode())
def check_network_status():
# 检查网络状态
cmd = "ifconfig"
output = subprocess.check_output(cmd, shell=True)
print("网络状态:")
print(output.decode())
def check_cpu_usage():
# 检查CPU使用情况
cmd = "top -n 1 -b | grep Cpu"
output = subprocess.check_output(cmd, shell=True)
print("CPU使用情况:")
print(output.decode())
def check_system_information():
# 检查系统信息
cmd = "uname -a"
output = subprocess.check_output(cmd, shell=True)
print("系统信息:")
print(output.decode())
def main():
# 调用各个检查函数
check_disk_usage()
check_memory_usage()
check_network_status()
check_cpu_usage()
check_system_information()
if __name__ == "__main__":
main()
```
这个脚本使用`subprocess`模块来执行命令行命令,并通过`print`语句来打印结果。你可以根据需要添加更多的检查函数或自定义脚本的其他功能。注意,这只是一个简单的示例脚本,实际情况可能需要更复杂或更专业的脚本来执行更详细的巡检任务。
阅读全文
相关推荐
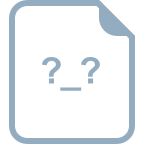
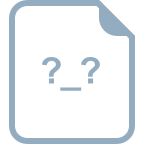
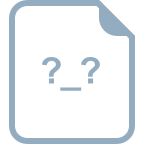
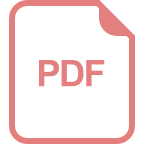
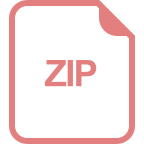
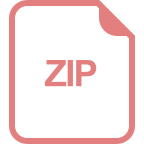
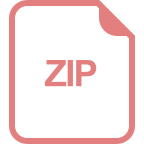