写一个方法:function2,参数为:文件路径名。 功能要求: ① 获取该路径下所有 Excel 文件(含子文件夹下),并把 Excel 文件最后修改时间为:一 周之内的 Excel 内容,合并到 mzy-merge.xlsx 文件中。 ② mzy-merge.xlsx 文件中,每个被合并文件内容最前部插入:该文件绝对路径,且背景色 为黄色,字体为红色。 ③ 返回值为:mzy-merge.xlsx 文件的绝对路径字符串
时间: 2024-01-22 15:17:57 浏览: 69
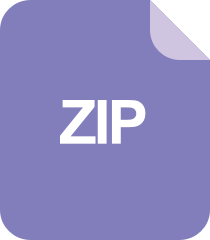
Excel-VBA宏编程实例源代码-获取上一级文件夹的路径.zip
以下是实现该方法的代码,注释中有详细的说明:
```javascript
const fs = require('fs');
const path = require('path');
const xlsx = require('xlsx');
function function2(dirPath) {
// 获取 mzy-merge.xlsx 文件的绝对路径
const mergeFilePath = path.join(dirPath, 'mzy-merge.xlsx');
// 如果 mzy-merge.xlsx 文件不存在,则新建一个工作簿
let workbook;
if (!fs.existsSync(mergeFilePath)) {
workbook = xlsx.utils.book_new();
} else {
// 否则读取已有的工作簿
const buffer = fs.readFileSync(mergeFilePath);
workbook = xlsx.read(buffer, {type: 'buffer'});
}
// 遍历该路径下所有文件和子文件夹
const files = fs.readdirSync(dirPath);
for (const file of files) {
const filePath = path.join(dirPath, file);
const stat = fs.statSync(filePath);
if (stat.isDirectory()) {
// 如果是文件夹,则递归调用 function2
function2(filePath);
} else if (stat.isFile()) {
// 如果是文件,并且是 Excel 文件,并且最后修改时间为一周之内
if (/\.xlsx?$/.test(file) && Date.now() - stat.mtime.getTime() <= 7 * 24 * 60 * 60 * 1000) {
// 读取 Excel 文件内容
const buffer = fs.readFileSync(filePath);
const worksheet = xlsx.read(buffer).Sheets[Object.keys(workbook.Sheets)[0]];
// 在 mzy-merge.xlsx 中新建一个工作表,把该文件内容合并到该工作表中
const sheetName = path.basename(filePath, path.extname(filePath));
xlsx.utils.book_append_sheet(workbook, worksheet, sheetName);
// 在该文件内容最前部插入该文件绝对路径,背景色为黄色,字体为红色
const range = xlsx.utils.decode_range(worksheet['!ref']);
const cellRef = xlsx.utils.encode_cell({r: range.s.r, c: range.s.c});
const cellValue = {v: filePath, s: {fill: {fgColor: {rgb: 'FFFF00'}}, font: {color: {rgb: 'FF0000'}}}};
worksheet[cellRef] = cellValue;
}
}
}
// 将修改后的工作簿保存到 mzy-merge.xlsx 文件中
const buffer = xlsx.write(workbook, {type: 'buffer'});
fs.writeFileSync(mergeFilePath, buffer);
return mergeFilePath;
}
```
使用方法:
```javascript
const mergeFilePath = function2('/path/to/dir');
console.log(mergeFilePath);
```
阅读全文
相关推荐
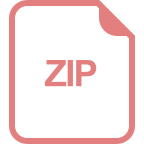
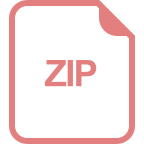

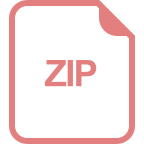
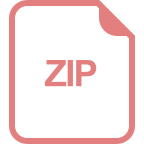
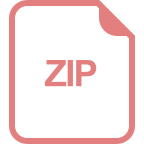
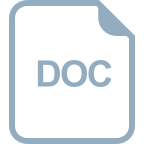
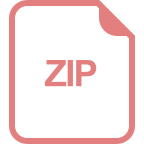
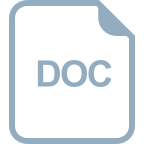
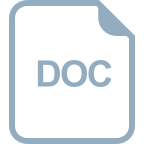



