C#但是回调函数中循化读取一部分队列中里面还有队列,但是代码不会等待直接往下执行其他代码了
时间: 2024-01-23 09:02:31 浏览: 107
在 RabbitMQ 中,消费者一般会使用 `BasicConsume` 方法来消费队列中的消息,这个方法是异步的,所以并不会等待消息的到来,而是在有消息到来时才会触发回调函数。
如果你想在回调函数中循环读取队列中的消息,可以在回调函数中使用 `while` 循环不断读取消息,然后在读取完消息后再进行下一步操作。
以下是一个示例代码,演示了如何在回调函数中循环读取队列中的消息:
```csharp
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System.Text;
public class RabbitMQConsumer
{
private readonly IConnection _connection;
private readonly IModel _channel;
public RabbitMQConsumer()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
_connection = factory.CreateConnection();
_channel = _connection.CreateModel();
// 声明队列
_channel.QueueDeclare(queue: "test_queue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
// 创建消费者
var consumer = new EventingBasicConsumer(_channel);
// 绑定消息接收事件
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
// 处理消息
while(true)
{
// 读取队列中的消息
var nextMessage = _channel.BasicGet("test_queue", false);
if(nextMessage == null)
{
// 队列中没有更多消息了,跳出循环
break;
}
// 处理消息
var nextBody = nextMessage.Body.ToArray();
var nextMessageText = Encoding.UTF8.GetString(nextBody);
// ...
}
// 在处理完队列中的消息后,进行下一步操作
// ...
};
// 开始消费消息
_channel.BasicConsume(queue: "test_queue",
autoAck: true,
consumer: consumer);
}
public void Close()
{
_channel.Close();
_connection.Close();
}
}
```
在上面的代码中,我们在回调函数中使用了一个 `while` 循环来读取队列中的消息。在每次循环中,我们使用 `_channel.BasicGet` 方法读取队列中的下一条消息,如果队列中没有更多消息了,就跳出循环。在处理完队列中的消息后,我们可以在回调函数中进行下一步操作。
阅读全文
相关推荐
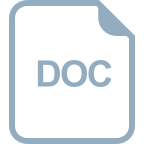
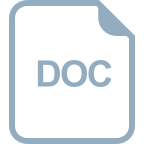
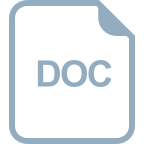
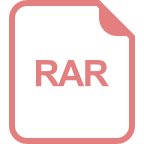
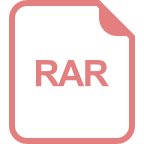
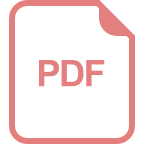
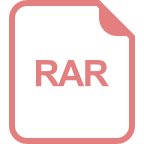
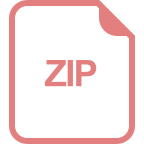
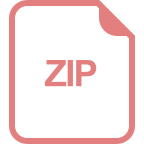
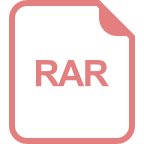
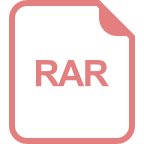
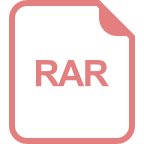
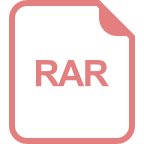
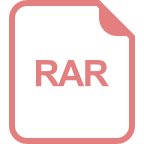
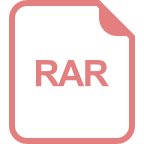
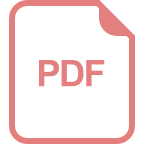
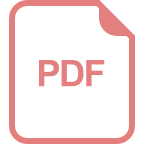
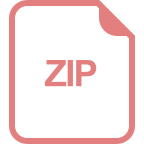
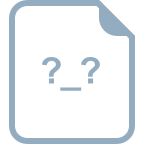