使用C语言写一个单片机的实时消息系统
时间: 2023-09-08 20:12:20 浏览: 56
实时消息系统通常使用队列和中断来实现。下面是一个简单的实时消息系统的C语言代码示例:
```c
#include <avr/io.h>
#include <avr/interrupt.h>
// 定义消息结构体
typedef struct {
uint8_t type; // 消息类型
uint8_t data[4]; // 消息数据
} Message;
// 定义消息队列
#define QUEUE_SIZE 16
volatile Message message_queue[QUEUE_SIZE];
volatile uint8_t queue_front = 0;
volatile uint8_t queue_rear = 0;
// 定义中断服务函数,接收串口数据,并将消息加入队列
ISR(USART_RX_vect) {
Message msg;
// 从串口接收数据,并填充消息结构体
msg.type = UDR0;
for (int i = 0; i < 4; i++) {
while (!(UCSR0A & (1 << RXC0)));
msg.data[i] = UDR0;
}
// 将消息加入队列
uint8_t next_rear = (queue_rear + 1) % QUEUE_SIZE;
if (next_rear != queue_front) {
message_queue[queue_rear] = msg;
queue_rear = next_rear;
}
}
// 定义任务结构体
typedef struct {
void (*task)(void); // 任务函数指针
uint16_t period; // 任务执行周期
uint16_t count; // 计数器,用于确定任务何时执行
} Task;
// 定义任务列表,包含1个任务
Task task_list[1] = {
{task1, 100, 0}
};
// 定义计时器计数器
volatile uint16_t timer_count = 0;
// 定义中断服务函数,每1ms执行一次
ISR(TIMER1_COMPA_vect) {
// 计时器计数器加1
timer_count++;
// 遍历任务列表,检查是否有任务需要执行
for (int i = 0; i < 1; i++) {
Task *task = &task_list[i];
if (timer_count % task->period == 0) {
// 如果计数器能够整除任务执行周期,说明任务需要执行
task->count++;
if (task->count == 1) {
// 如果是第一次执行任务,直接调用任务函数
task->task();
} else if (task->count >= task->period / 1000) {
// 如果计数器达到了任务执行周期,则重置计数器,并执行任务函数
task->count = 0;
task->task();
}
}
}
}
int main(void) {
// 初始化串口,设置波特率为9600
UBRR0H = 0;
UBRR0L = 103;
UCSR0B |= (1 << RXEN0) | (1 << RXCIE0);
UCSR0C |= (1 << UCSZ01) | (1 << UCSZ00);
// 初始化定时器1,设置为CTC模式,频率为1MHz,触发中断的时间间隔为1ms
TCCR1B |= (1 << WGM12) | (1 << CS10);
OCR1A = 999;
TIMSK1 |= (1 << OCIE1A);
// 开启全局中断
sei();
while (1) {
// 处理消息队列中的消息
while (queue_front != queue_rear) {
Message msg = message_queue[queue_front];
queue_front = (queue_front + 1) % QUEUE_SIZE;
// 根据消息类型执行相应的操作
if (msg.type == 0x01) {
// 执行操作1
} else if (msg.type == 0x02) {
// 执行操作2
}
}
}
return 0;
}
// 示例任务函数
void task1(void) {
// 发送一条消息到串口
Message msg;
msg.type = 0x01;
msg.data[0] = 0x11;
msg.data[1] = 0x22;
msg.data[2] = 0x33;
msg.data[3] = 0x44;
while (!(UCSR0A & (1 << UDRE0)));
UDR0 = msg.type;
for (int i = 0; i < 4; i++) {
while (!(UCSR0A & (1 << UDRE0)));
UDR0 = msg.data[i];
}
}
```
这个实时消息系统使用串口接收中断来接收消息,并将消息加入队列。主循环中处理消息队列中的消息,根据消息类型执行相应的操作。这个实时消息系统可以接收任意类型的消息,并且可以非常方便地扩展支持更多类型的消息。同时,这个实时消息系统也可以执行多个任务,并且每个任务的执行周期可以自由设置。
相关推荐
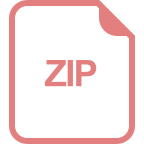
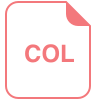
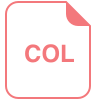
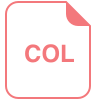
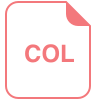
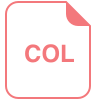









