写一段python代码实现文档之间查重需要
时间: 2024-01-29 11:03:15 浏览: 81
先进行文本预处理,包括去除停用词、特殊符号、数字等,对文本进行分词并进行词性标注和去除标点符号。然后利用TF-IDF算法计算文本相似度,通过设置阈值来判断两篇文档是否相似。具体实现代码如下:
```python
import jieba
import jieba.analyse
import jieba.posseg as pseg
import math
def preprocess(text):
"""
对文本进行预处理,包括去除停用词、特殊符号、数字等
"""
# 去除特殊符号和数字
text = re.sub('[^\\u4e00-\\u9fa5a-zA-Z]', '', text)
# 分词并去除停用词
words = [w.word for w in pseg.cut(text) if w.flag[0] not in ['x', 'u', 'w'] and w.word not in stopwords]
return words
def get_tf(words):
"""
计算单词的词频
"""
tf = {}
for word in words:
if word not in tf:
tf[word] = 0
tf[word] += 1
return tf
def get_idf(words, idf_dict):
"""
计算单词的逆文档频率
"""
idf = {}
for word in words:
if word not in idf:
idf[word] = math.log(len(idf_dict) / (idf_dict.get(word, 0) + 1))
return idf
def get_tfidf(tf, idf):
"""
计算单词的TF-IDF值
"""
tfidf = {}
for word in tf:
tfidf[word] = tf[word] * idf[word]
return tfidf
def get_similarity(tfidf1, tfidf2):
"""
计算两个文档的相似度
"""
numerator = 0
denominator1 = 0
denominator2 = 0
for word in tfidf1:
numerator += tfidf1[word] * tfidf2.get(word, 0)
denominator1 += tfidf1[word] ** 2
for word in tfidf2:
denominator2 += tfidf2[word] ** 2
denominator = math.sqrt(denominator1) * math.sqrt(denominator2)
if denominator == 0:
return 0
else:
return numerator / denominator
def is_duplicate(text1, text2, threshold=0.8):
"""
判断两篇文档是否相似
"""
words1 = preprocess(text1)
words2 = preprocess(text2)
tf1 = get_tf(words1)
tf2 = get_tf(words2)
idf_dict = dict.fromkeys(set(words1 + words2), 0)
idf_dict.update(get_idf(words1, idf_dict))
idf_dict.update(get_idf(words2, idf_dict))
tfidf1 = get_tfidf(tf1, idf_dict)
tfidf2 = get_tfidf(tf2, idf_dict)
similarity = get_similarity(tfidf1, tfidf2)
if similarity >= threshold:
return True
else:
return False
```
调用该函数可以判断两篇文档是否相似,例如:
```python
text1 = "这是一篇测试文档,用于测试相似度计算。"
text2 = "这篇文档是用来测试相似度计算的。"
if is_duplicate(text1, text2):
print("两篇文档相似")
else:
print("两篇文档不相似")
```
阅读全文
相关推荐
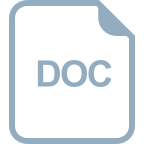
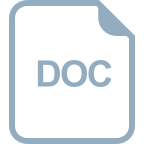
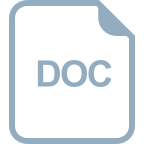
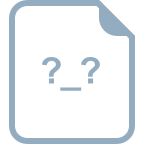
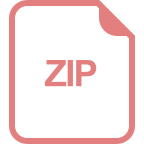
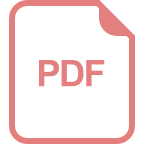
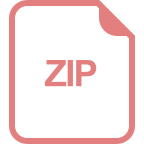
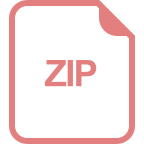
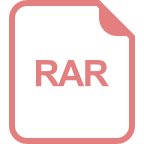
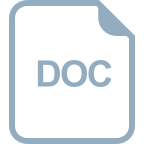
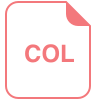
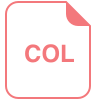

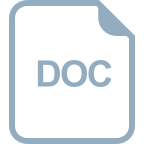
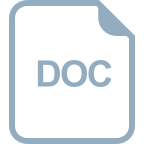
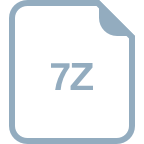
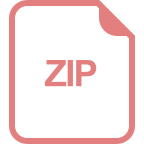
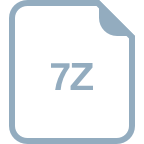