补全cancelThread函数,使用pthread_cancel函数终止指定的线程,thread为线程要被取消的线程ID号,调用成功返回0,否则返回-1
时间: 2023-08-16 22:08:41 浏览: 204
下面是补全的代码:
```c
int cancelThread(pthread_t thread) {
int ret = pthread_cancel(thread);
if (ret != 0) {
perror("pthread_cancel");
return -1;
}
return 0;
}
```
该函数接收一个线程ID号,使用pthread_cancel函数终止指定的线程。如果调用成功返回0,否则返回-1。在函数内部对返回值进行了判断,如果返回值不为0则输出错误信息,并返回-1。
相关问题
补全waitThread函数,使用pthread_join函数挂起当前线程,等待指定线程结束,thread为要等待的线程ID号,waitThread函数等待线程成功返回0,失败返回-1
下面是补全后的waitThread函数代码:
```
int waitThread(pthread_t thread) {
int ret = pthread_join(thread, NULL);
if (ret != 0) {
return -1;
}
return 0;
}
```
在该函数中,我们使用pthread_join函数来挂起当前线程,等待指定线程结束。如果成功等待到了指定线程的结束,pthread_join函数将返回0,我们将其返回值作为waitThread函数的返回值。如果等待失败,pthread_join函数将返回一个非零值,我们将其转换为-1返回。
补全createThread函数,使用pthread_create函数创建线程,并将start_routine作为线程处理函数,arg作为线程处理函数的参数,同时将创建成功的线程ID作为createThread函数的返回值
在C语言中,我们可以使用POSIX线程库(pthread)来创建线程。下面是一个补全`createThread`函数的例子,它会使用`pthread_create`函数来创建一个新的线程:
```c
#include <pthread.h>
// 假设 start_routine 是线程处理函数,arg 是传递给它的参数
typedef void (*thread_func)(void* arg);
int createThread(thread_func start_routine, void* arg) {
pthread_t thread_id; // 线程ID变量
int result;
// 创建线程
result = pthread_create(&thread_id, NULL, start_routine, arg);
if (result != 0) {
printf("Error creating thread: %d\n", result);
return -1; // 返回错误码
}
// 如果创建成功,返回线程ID
printf("Thread created with ID: %ld\n", (long)thread_id);
return thread_id;
}
// 示例线程处理函数
void my_thread_function(void* data) {
// 这里是对数据data的操作
printf("Thread function running with argument: %p\n", data);
}
int main() {
int some_data = 42;
// 调用createThread并传入函数和参数
pthread_t new_thread = createThread(my_thread_function, &some_data);
if (new_thread == -1) {
// 处理创建失败的情况
exit(1);
}
// 主线程继续执行其他任务...
pthread_join(new_thread, NULL); // 等待新线程结束
return 0;
}
```
在这个示例中,`createThread`函数接受一个指向`thread_func`类型的指针以及一个用户数据指针`arg`,然后使用`pthread_create`创建线程。如果创建成功,函数返回新创建线程的ID;若失败,则返回错误代码。
阅读全文
相关推荐














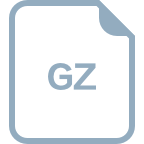
