如何定位recyclerview的adapter的position
时间: 2024-04-02 11:36:41 浏览: 16
RecyclerView的Adapter中的position可以通过ViewHolder中的getAdapterPosition()方法来获取,该方法会返回当前ViewHolder所对应的数据在Adapter中的位置,如果ViewHolder没有绑定数据,那么该方法会返回RecyclerView.NO_POSITION。需要注意的是,由于RecyclerView的机制是在屏幕上显示的Item View以及其上、下方各多预留一些Item View,因此同一个Item View会在多个位置上出现,从而导致position重复。如果需要在RecyclerView中对Item View进行定位,可以结合RecyclerView.LayoutManager的方法来实现,例如LinearLayoutManager的findFirstVisibleItemPosition()和findLastVisibleItemPosition()方法可以分别返回当前屏幕上第一个可见Item View和最后一个可见Item View在Adapter中的位置。
相关问题
android kotlin RecyclerView adapter
RecyclerView is a UI component that is used to display large sets of data in an efficient and scrollable manner. The RecyclerView widget is a more advanced and flexible version of ListView. To use RecyclerView, you need to create an adapter that will hold the data and create the views to be displayed. Here is an example of a RecyclerView adapter in Kotlin:
```
class MyAdapter(private val dataList: List<MyData>) : RecyclerView.Adapter<MyAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
val data = dataList[position]
holder.bind(data)
}
override fun getItemCount() = dataList.size
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
fun bind(data: MyData) {
// bind data to views
itemView.textViewTitle.text = data.title
itemView.textViewDescription.text = data.description
}
}
}
```
In this example, `MyData` is a data class that holds the data to be displayed in the RecyclerView. The `MyAdapter` class takes a list of `MyData` objects as a parameter in the constructor. The `onCreateViewHolder` method inflates a layout file (`item_layout.xml` in this case) and returns a `ViewHolder` object. The `onBindViewHolder` method binds the data to the views in the `ViewHolder`. Finally, the `getItemCount` method returns the number of items in the list, which is the size of the `dataList`. The `ViewHolder` class holds the views that will be displayed in the RecyclerView and the `bind` method binds the data to the views.
recyclerview viewholder adapter使用
RecyclerView是Android提供的一个非常灵活的控件,用于显示大量数据的列表,它的核心是ViewHolder和Adapter。
ViewHolder是RecyclerView的一个子类,它主要用于缓存item的视图,以便能够快速地滚动和重新使用这些视图。ViewHolder的实现非常简单,只需要继承RecyclerView.ViewHolder,然后在构造函数中初始化视图,最后将视图绑定到ViewHolder中。
Adapter是RecyclerView的另一个子类,它主要用于管理数据和视图之间的关系。Adapter必须实现三个方法:onCreateViewHolder、onBindViewHolder和getItemCount。其中onCreateViewHolder方法用于创建ViewHolder,onBindViewHolder方法用于将数据绑定到ViewHolder中,getItemCount方法用于获取数据的数量。
以下是一个简单的RecyclerView、ViewHolder和Adapter的实现示例:
ViewHolder的实现:
```
public class MyViewHolder extends RecyclerView.ViewHolder {
private TextView mTextView;
public MyViewHolder(View itemView) {
super(itemView);
mTextView = itemView.findViewById(R.id.text_view);
}
public void bind(String text) {
mTextView.setText(text);
}
}
```
Adapter的实现:
```
public class MyAdapter extends RecyclerView.Adapter<MyViewHolder> {
private List<String> mData;
public MyAdapter(List<String> data) {
mData = data;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.item_view, parent, false);
return new MyViewHolder(itemView);
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.bind(mData.get(position));
}
@Override
public int getItemCount() {
return mData.size();
}
}
```
在这个示例中,我们创建了一个ViewHolder,它包含一个TextView,用于显示列表项的文本。我们还创建了一个Adapter,它管理一个字符串列表,并将每个字符串绑定到ViewHolder中的TextView中。
最后,我们需要将Adapter设置到RecyclerView中:
```
RecyclerView recyclerView = findViewById(R.id.recycler_view);
MyAdapter adapter = new MyAdapter(data);
recyclerView.setAdapter(adapter);
```
这样就可以显示一个简单的RecyclerView了。当我们滚动列表时,RecyclerView会自动回收不再需要的视图,并将它们重新用于新的列表项。
相关推荐
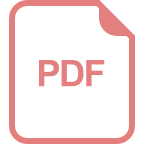
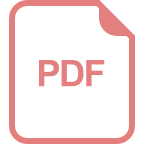
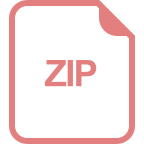












