要求从Student类中创建对象后调用计算面积的方法,方法参数为圆形类对象时计算圆形面积,参数为梯形类对象时计算梯形面积。
时间: 2024-06-13 08:09:24 浏览: 44
以下是Java代码示例,实现从Student类中创建对象后调用计算面积的方法,方法参数为圆形类对象时计算圆形面积,参数为梯形类对象时计算梯形面积:
```java
public class Student {
private int id;
private String name;
private int age;
public Student(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public double calculateArea(Shape shape) {
if (shape instanceof Circle) {
Circle circle = (Circle) shape;
return circle.getRadius() * circle.getRadius() * Math.PI;
} else if (shape instanceof Trapezoid) {
Trapezoid trapezoid = (Trapezoid) shape;
return (trapezoid.getTop() + trapezoid.getBottom()) * trapezoid.getHeight() / 2;
} else {
return 0;
}
}
}
abstract class Shape {
public abstract double getArea();
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return radius * radius * Math.PI;
}
}
class Trapezoid extends Shape {
private double top;
private double bottom;
private double height;
public Trapezoid(double top, double bottom, double height) {
this.top = top;
this.bottom = bottom;
this.height = height;
}
public double getTop() {
return top;
}
public void setTop(double top) {
this.top = top;
}
public double getBottom() {
return bottom;
}
public void setBottom(double bottom) {
this.bottom = bottom;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public double getArea() {
return (top + bottom) * height / 2;
}
}
// 创建Student对象并调用calculateArea方法
Student student = new Student(1, "Tom", 18);
Circle circle = new Circle(5);
Trapezoid trapezoid = new Trapezoid(3, 5, 4);
double circleArea = student.calculateArea(circle);
double trapezoidArea = student.calculateArea(trapezoid);
System.out.println("圆形面积为:" + circleArea);
System.out.println("梯形面积为:" + trapezoidArea);
```
阅读全文
相关推荐
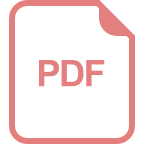
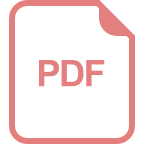
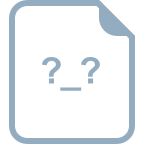
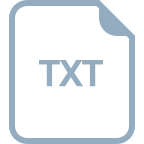
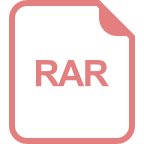
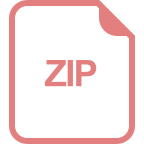
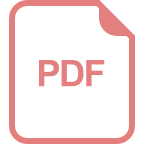
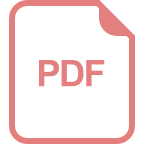
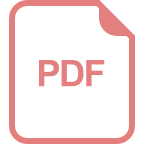
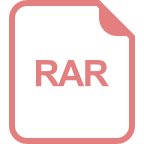
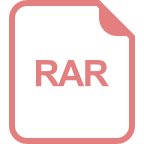
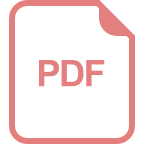
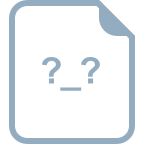
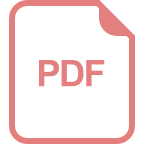
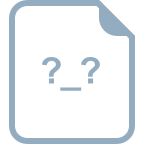
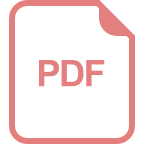
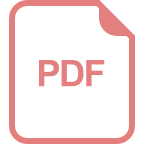