在c语言中用指针和数组实现输入一个年份和天数计算出第几月第几天
时间: 2025-01-01 14:39:21 浏览: 4
在 C 语言中,你可以通过指针和数组来实现这样的功能。首先,你需要两个变量,一个存储年份(整型 int),另一个存储总天数(也应该是整型)。假设用户输入的是从年初到现在的天数,你需要考虑每个月的天数差异,包括闰年的处理。
下面是一个简单的示例程序:
```c
#include <stdio.h>
int main() {
int year, total_days;
printf("请输入年份: ");
scanf("%d", &year);
printf("请输入总天数: ");
scanf("%d", &total_days);
// 定义月份和天数数组,注意二月需要特殊处理闰年的情况
int days_in_month[] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int current_month = 1; // 当前月份,默认为1月
for (int i = 0; i < total_days; ++i) {
if (current_month == 2 && is_leap_year(year)) {
days_in_month[2] = 29; // 闰年二月有29天
}
if (days_in_month[current_month - 1] <= i) {
i -= days_in_month[current_month - 1];
current_month++;
} else {
break;
}
}
printf("第%d个月第%d天\n", current_month, i + 1); // 加1是因为我们是从0开始计数的
return 0;
}
// 辅助函数判断是否为闰年
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 != 0)
return false;
else
return true;
}
```
在这个程序中,我们遍历每一天,直到达到指定的总天数,然后记录当前月份。注意闰年的处理是在`is_leap_year`辅助函数中完成的。这个实现假设用户只输入了一个自然年内的天数,并且忽略了一年中的其他特殊情况,比如连续闰年等。
阅读全文
相关推荐




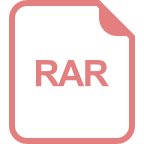

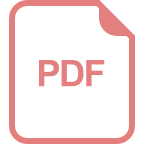








