青年歌手参加歌曲大奖赛,有8个评委对他进行打分,试使用数组知识编程求这位选手的平均得分(去掉一个最高分和一个最低分,小数点保留两位)c语言
时间: 2024-12-22 21:18:55 浏览: 6
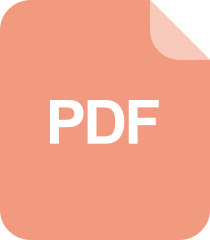
C语言实现歌手大奖赛计分程序

在C语言中,你可以使用一个动态数组来存储评委的打分,然后通过循环遍历数组,找出最高分和最低分并去除,最后计算剩余得分的平均值。以下是代码的一个示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h> // 引入数学库用于浮点数的处理
// 结构体定义评委打分
typedef struct {
int score;
} JudgeScore;
// 计算平均得分
double calculateAverage(JudgeScore scores[], int size) {
if (size <= 1) {
printf("至少需要两个分数才能计算平均分。\n");
return -1; // 返回错误值,表示无法计算
}
// 排序数组
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (scores[j].score > scores[j + 1].score) {
JudgeScore temp = scores[j];
scores[j] = scores[j + 1];
scores[j + 1] = temp;
}
}
}
// 找到最高分和最低分,并排除
int highestIndex = 0;
int lowestIndex = size - 1;
double sumWithoutMinMax = 0;
for (int i = 1; i < size - 1; i++) {
sumWithoutMinMax += scores[i].score;
}
// 计算平均分并保留两位小数
double average = sumWithoutMinMax / (size - 2);
return round(average * 100) / 100.0; // 使用round四舍五入到小数点后两位
}
int main() {
// 假设有8位评委打分
JudgeScore judgeScores[8];
int size;
printf("请输入评委对青年歌手的评分(共8个):\n");
for (size = 0; size < 8; size++) {
scanf("%d", &judgeScores[size].score);
}
double avgScore = calculateAverage(judgeScores, size);
if (avgScore != -1) {
printf("青年歌手的平均得分(去掉了最高分和最低分)为: %.2f\n", avgScore);
}
return 0;
}
```
这个程序首先读取评委的打分,然后排序数组、找到最高分和最低分并剔除,最后计算剩余分数的平均值。注意,如果只收到一个或没有分数,程序会提示错误并返回 `-1`。
阅读全文
相关推荐
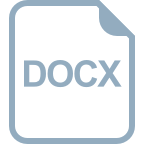
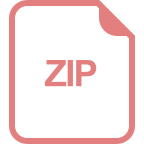










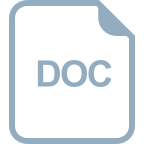
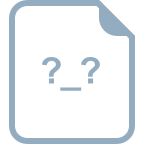