学籍管理系统c语言链表
时间: 2024-12-27 10:19:45 浏览: 0
### C语言实现学籍管理系统的链表示例
#### 创建节点结构体
为了有效地管理和操作学生数据,定义一个`struct`来表示链表中的每一个节点。这个结构体不仅包含学生的具体信息还含有指向下一个节点的指针。
```c
#include <stdio.h>
#include <stdlib.h> // For malloc()
// Define the structure of a node in our linked list.
typedef struct Student {
char name[50];
int id;
float score;
struct Student *pNext;
} Node;
```
#### 初始化头结点
创建一个新的链表时,通常会先初始化一个空的头部节点作为起点。
```c
Node* InitializeList(void) {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) exit(-1);
head->pNext = NULL;
return head;
}
```
#### 添加新记录到链表末端
当有新的学生加入学校时,在链表最后追加一条新的记录是很常见的需求之一。
```c
void AddNewRecord(Node **ppHead, const char *name, int id, float score) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->id = id;
strcpy(newNode->name, name);
newNode->score = score;
newNode->pNext = NULL;
Node *current = *ppHead;
while(current->pNext != NULL){
current = current->pNext;
}
current->pNext = newNode;
}
```
#### 显示所有学生的信息
遍历整个链表并打印出每个学生的名字、ID以及成绩等基本信息。
```c
void DisplayAllRecords(const Node *pHead) {
puts("显示全部学生信息:");
for(pHead=pHead->pNext; pHead!=NULL; pHead=pHead->pNext){
printf("%s\t%d\t%.2f\n", pHead->name, pHead->id, pHead->score);
}
}
```
#### 统计所有学生的人数
通过遍历链表计算当前注册了多少名学生[^3]。
```c
int CountStudents(const Node *pHead) {
int countStu = 0;
while (pHead->pNext != NULL) {
++countStu;
pHead = pHead->pNext;
}
return countStu;
}
```
以上就是基于C语言使用单向链表构建简单的学生信息系统的主要功能模块。这些函数可以组合起来形成更复杂的应用程序逻辑,比如允许用户输入命令来进行增删改查等操作。
阅读全文
相关推荐
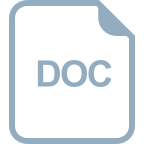
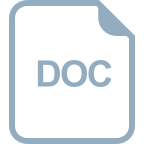
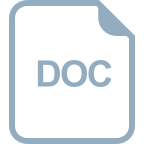
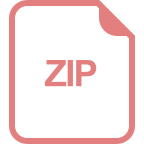
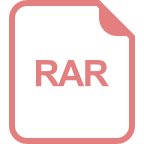
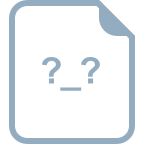
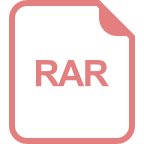
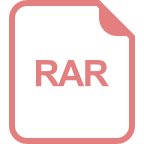
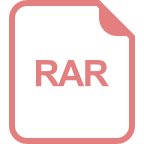



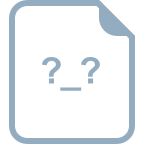
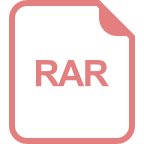
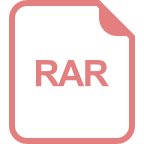
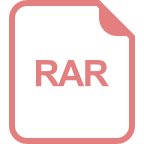
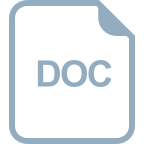
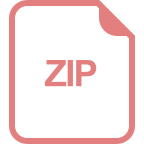