请简述Spring Boot使用注解方式整个Mybatis访问数据库步骤
时间: 2024-03-19 13:41:35 浏览: 54
首先,需要在pom.xml文件中添加mybatis-spring-boot-starter依赖。然后,创建一个Mapper接口,并使用@Mapper注解进行标注。在Mapper接口中,可以定义SQL语句的方法。接下来,需要在application.properties文件中配置数据源和Mybatis的相关属性,例如数据库连接信息、Mapper接口的包名等。最后,在Spring Boot的启动类上使用@MapperScan注解,指定Mapper接口的包名,这样Spring Boot就可以自动扫描并注入Mapper接口了。这样,就完成了使用注解方式整合Mybatis访问数据库的步骤。
相关问题
请简述spring boot使用注解方式整合mybatis访问数据库步骤
首先,需要在pom.xml文件中添加mybatis-spring-boot-starter依赖。然后,创建一个Mapper接口,并使用@Mapper注解进行标注。在Mapper接口中,可以定义SQL语句的方法。接下来,需要在application.properties文件中配置数据源和Mybatis的相关属性,例如数据库连接信息、Mapper接口的包名等。最后,在Spring Boot的启动类上使用@MapperScan注解,指定Mapper接口的包名,这样Spring Boot就可以自动扫描并注入Mapper接口了。这样,就完成了使用注解方式整合Mybatis访问数据库的步骤。
spring boot使用注解整合mybatis
Spring Boot整合MyBatis可以使用`@MapperScan`注解来扫描Mapper接口,同时需要在配置文件中配置MyBatis的相关信息。
1. 添加依赖
在`pom.xml`中添加MyBatis和MyBatis-SpringBoot-Starter依赖:
```
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>${mybatis.version}</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>${mybatis.version}</version>
</dependency>
```
其中`${mybatis.version}`表示MyBatis的版本号。
2. 配置数据源
在`application.properties`中配置数据源相关信息,这里以MySQL为例:
```
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
```
3. 配置MyBatis
在`application.properties`中配置MyBatis相关信息:
```
# mybatis
mybatis.type-aliases-package=com.example.demo.entity
mybatis.mapper-locations=classpath:mapper/*.xml
```
其中`mybatis.type-aliases-package`表示实体类所在的包,`mybatis.mapper-locations`表示Mapper接口对应的XML文件所在的位置。
4. 编写Mapper接口和XML文件
编写Mapper接口和对应的XML文件,这里以`UserMapper`为例:
```
@Mapper
public interface UserMapper {
User findById(Long id);
}
```
```
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
<select id="findById" parameterType="long" resultType="com.example.demo.entity.User">
select * from user where id = #{id}
</select>
</mapper>
```
5. 使用注解扫描Mapper接口
在启动类上使用`@MapperScan`注解扫描Mapper接口所在的包:
```
@SpringBootApplication
@MapperScan("com.example.demo.mapper")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
6. 测试
在Controller中注入Mapper接口,并调用方法测试是否能够正常访问数据库:
```
@RestController
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/user/{id}")
public User getUserById(@PathVariable Long id) {
return userMapper.findById(id);
}
}
```
访问`/user/1`可以获取到id为1的用户信息。
阅读全文
相关推荐
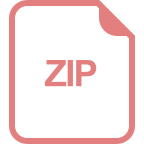
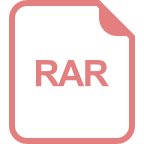
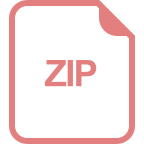













