用C++设计一个通讯录管理系统,并指出其中的数据结构
时间: 2024-05-07 19:23:08 浏览: 137
一个通讯录管理系统可以使用以下数据结构:
1. 结构体(struct):用于存储每个联系人的信息,包括姓名、电话号码、地址等。
2. 动态数组(vector):用于存储所有联系人的结构体,可以方便地进行添加、删除、修改等操作。
3. 链表(linked list):用于实现联系人的排序和查找功能,可以根据姓名首字母或者其他字段进行排序,并且可以快速查找指定联系人。
4. 文件(file):用于将联系人信息保存到磁盘上,保证数据的持久化。
以下是一个简单的通讯录管理系统的 C++ 代码示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <fstream>
using namespace std;
struct Contact {
string name;
string phone_number;
string address;
};
vector<Contact> contacts;
void add_contact() {
Contact contact;
cout << "Enter name: ";
getline(cin, contact.name);
cout << "Enter phone number: ";
getline(cin, contact.phone_number);
cout << "Enter address: ";
getline(cin, contact.address);
contacts.push_back(contact);
}
void delete_contact() {
string name;
cout << "Enter name to delete: ";
getline(cin, name);
for (auto it = contacts.begin(); it != contacts.end(); it++) {
if (it->name == name) {
contacts.erase(it);
cout << "Contact deleted." << endl;
return;
}
}
cout << "Contact not found." << endl;
}
void modify_contact() {
string name;
cout << "Enter name to modify: ";
getline(cin, name);
for (auto& contact : contacts) {
if (contact.name == name) {
cout << "Enter new phone number: ";
getline(cin, contact.phone_number);
cout << "Enter new address: ";
getline(cin, contact.address);
cout << "Contact modified." << endl;
return;
}
}
cout << "Contact not found." << endl;
}
void search_contact() {
string name;
cout << "Enter name to search: ";
getline(cin, name);
for (auto& contact : contacts) {
if (contact.name == name) {
cout << "Name: " << contact.name << endl;
cout << "Phone number: " << contact.phone_number << endl;
cout << "Address: " << contact.address << endl;
return;
}
}
cout << "Contact not found." << endl;
}
void list_contacts() {
sort(contacts.begin(), contacts.end(), [](const Contact& a, const Contact& b) {
return a.name < b.name;
});
for (const auto& contact : contacts) {
cout << "Name: " << contact.name << endl;
cout << "Phone number: " << contact.phone_number << endl;
cout << "Address: " << contact.address << endl;
cout << endl;
}
}
void save_contacts() {
ofstream file("contacts.txt");
for (const auto& contact : contacts) {
file << contact.name << "," << contact.phone_number << "," << contact.address << endl;
}
file.close();
cout << "Contacts saved." << endl;
}
void load_contacts() {
ifstream file("contacts.txt");
if (file.is_open()) {
string line;
while (getline(file, line)) {
Contact contact;
size_t pos = line.find(",");
contact.name = line.substr(0, pos);
line.erase(0, pos + 1);
pos = line.find(",");
contact.phone_number = line.substr(0, pos);
line.erase(0, pos + 1);
contact.address = line;
contacts.push_back(contact);
}
file.close();
cout << "Contacts loaded." << endl;
} else {
cout << "Unable to open file." << endl;
}
}
int main() {
load_contacts();
while (true) {
cout << "1. Add contact" << endl;
cout << "2. Delete contact" << endl;
cout << "3. Modify contact" << endl;
cout << "4. Search contact" << endl;
cout << "5. List contacts" << endl;
cout << "6. Save contacts" << endl;
cout << "7. Exit" << endl;
cout << "Enter your choice: ";
int choice;
cin >> choice;
cin.ignore();
switch (choice) {
case 1:
add_contact();
break;
case 2:
delete_contact();
break;
case 3:
modify_contact();
break;
case 4:
search_contact();
break;
case 5:
list_contacts();
break;
case 6:
save_contacts();
break;
case 7:
return 0;
default:
cout << "Invalid choice." << endl;
break;
}
cout << endl;
}
return 0;
}
```
这个程序使用了结构体、动态数组、文件以及字符串处理等多种数据结构和技术,可以实现基本的通讯录管理功能。
阅读全文
相关推荐
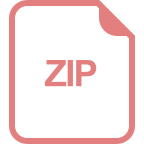
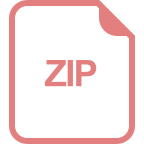
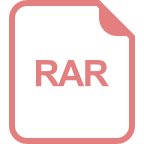
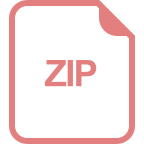
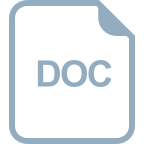
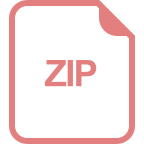
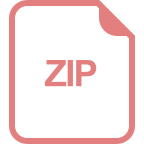
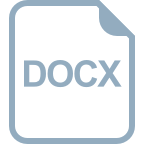
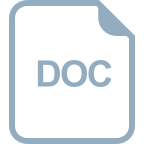
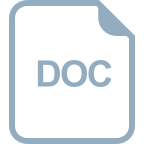
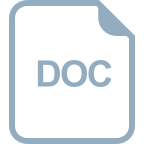
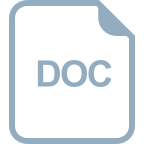
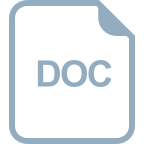
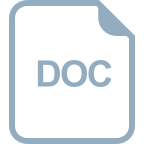
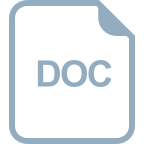
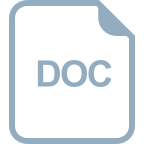
