如何用较为基础的c++语言编写上面那个程序
时间: 2024-03-10 13:46:03 浏览: 54
对于基础的 C++ 语言,实现一个类似于 ChitGPT 的 AI 对话程序需要进行以下步骤:
1. 定义一个变量来存储对话历史记录,以便 AI 能够了解上下文。
```
std::vector<std::string> history;
```
2. 定义一个函数来接收用户输入,并将其添加到历史记录中。
```
void add_to_history(std::string input) {
history.push_back(input);
}
```
3. 定义一个函数来生成 AI 的回复,可以使用随机数生成器或者基于规则的方法。
```
std::string generate_response() {
// 从历史记录中获取上一个输入
std::string last_input = history.back();
// 生成一个随机数,用于选择回复
int response_index = rand() % responses.size();
// 返回对应的回复
return responses[response_index];
}
```
4. 在主函数中循环接收用户输入,并调用以上函数来生成回复。
```
int main() {
// 初始化随机数生成器
srand(time(NULL));
// 循环接收用户输入
while (true) {
std::string input;
std::cout << "> ";
std::getline(std::cin, input);
// 将用户输入添加到历史记录中
add_to_history(input);
// 生成 AI 的回复并输出
std::string response = generate_response();
std::cout << "AI: " << response << std::endl;
}
return 0;
}
```
需要注意的是,以上代码只是基于 C++ 的简单实现,无法达到 ChitGPT 的复杂度和功能。如果需要实现更为复杂的 AI 对话程序,需要使用更为高级的算法和技术。
阅读全文
相关推荐



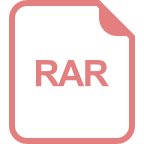
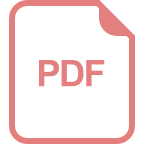










