基于python使用摄像头识别二维码
时间: 2024-05-06 15:19:34 浏览: 13
要使用Python识别二维码,我们需要安装以下库:
1. OpenCV:用于捕获视频并进行图像处理。
2. pyzbar:用于解码二维码。
在安装完这两个库之后,我们可以通过以下代码使用摄像头识别二维码:
```python
import cv2
from pyzbar import pyzbar
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头视频流
ret, frame = cap.read()
# 使用pyzbar库解码二维码
decoded_objs = pyzbar.decode(frame)
# 遍历所有解码出的二维码
for decoded_obj in decoded_objs:
# 提取二维码的位置和内容
x, y, w, h = decoded_obj.rect
barcode_data = decoded_obj.data.decode("utf-8")
barcode_type = decoded_obj.type
# 在图像上绘制矩形和文本
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
text = "{} ({})".format(barcode_data, barcode_type)
cv2.putText(frame, text, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示图像
cv2.imshow("Barcode Scanner", frame)
# 等待按下ESC键退出程序
if cv2.waitKey(1) == 27:
break
# 关闭摄像头
cap.release()
# 关闭显示窗口
cv2.destroyAllWindows()
```
在代码中,我们首先打开了摄像头,并在一个while循环中不断读取摄像头视频流。然后使用pyzbar库解码所有二维码,并在图像上绘制矩形和文本。最后,我们使用cv2.imshow()函数显示图像,并等待退出程序。
相关推荐
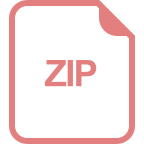














