vue3setup写一个有分页的表格代码
时间: 2024-05-12 07:16:16 浏览: 117
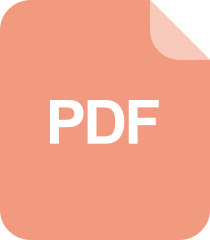
vue.js表格分页示例
好的,我会为您提供相关的代码。
首先,我们需要使用Vue 3框架,以及Bootstrap或Element UI等库来进行表格的展示和分页功能的实现。
在Vue 3中,我们可以使用Composition API来编写代码,使用setup函数来初始化组件,并在其中创建数据和方法。
下面是一个基本的有分页功能的表格的代码示例:
```
<template>
<div>
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in tableData.slice(startIndex, endIndex)" :key="index">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
</tr>
</tbody>
</table>
<nav>
<ul class="pagination justify-content-center">
<li class="page-item" :class="{ disabled: currentPage === 1 }">
<a class="page-link" href="#" @click.prevent="currentPage > 1 && (currentPage--);">
Previous
</a>
</li>
<li class="page-item" v-for="n in totalPage" :key="n" :class="{ active: currentPage === n }">
<a class="page-link" href="#" @click.prevent="currentPage = n;">{{ n }}</a>
</li>
<li class="page-item" :class="{ disabled: currentPage === totalPage }">
<a class="page-link" href="#" @click.prevent="currentPage < totalPage && (currentPage++);">
Next
</a>
</li>
</ul>
</nav>
</div>
</template>
<script>
import { reactive, computed } from 'vue'
export default {
name: 'TableComponent',
props: {
tableData: {
type: Array,
required: true
},
perPage: {
type: Number,
default: 10
}
},
setup (props) {
const state = reactive({
currentPage: 1
})
const totalPage = computed(() => Math.ceil(props.tableData.length / props.perPage))
const startIndex = computed(() => (state.currentPage - 1) * props.perPage)
const endIndex = computed(() => state.currentPage * props.perPage)
return {
state,
totalPage,
startIndex,
endIndex
}
}
}
</script>
```
在这个示例中,我们传入一个数组tableData作为表格数据,perPage作为每页显示的数据数量。使用props传入这些参数。
在setup函数中,我们使用reactive函数创建一个响应式对象state,其中包括currentPage属性,表示当前页码。
使用computed函数计算出totalPage总页数,startIndex当前页码对应的数据的起始下标,endIndex当前页码对应的数据的结束下标。
在template中使用v-for遍历数据,并根据当前页码和perPage计算出要显示的数据范围。
同时使用Bootstrap的分页组件,根据currentPage和totalPage生成分页按钮,通过@click.prevent来监听分页按钮的点击事件,根据点击的按钮来计算出新的currentPage并重新渲染页面。
希望这个示例代码对您有所帮助。
阅读全文
相关推荐




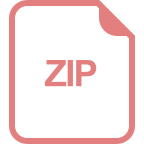









