帮我写一个c语言的程序,是一个通讯录,尝试将所有人员及其号码插入到哈希表结构中,并且带有一个search函数,这个函数可以搜索这个哈希表中的任意一个人
时间: 2023-04-08 12:03:19 浏览: 95
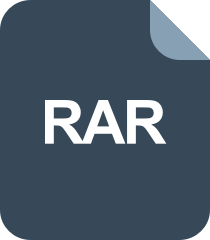
用C语言做的一个通讯录程序
好的,这是一个很好的问题。以下是一个简单的C语言程序,可以实现一个通讯录,使用哈希表来存储人员及其号码,并且带有一个search函数,可以搜索哈希表中的任意一个人。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 1000
typedef struct {
char name[50];
char phone[20];
} Person;
typedef struct {
Person *data;
int size;
} Bucket;
typedef struct {
Bucket *buckets;
int size;
} HashTable;
int hash(char *str) {
int hash = 0;
for (int i = 0; i < strlen(str); i++) {
hash += str[i];
}
return hash % TABLE_SIZE;
}
void initHashTable(HashTable *table) {
table->buckets = (Bucket*) malloc(TABLE_SIZE * sizeof(Bucket));
table->size = TABLE_SIZE;
for (int i = 0; i < table->size; i++) {
table->buckets[i].data = NULL;
table->buckets[i].size = 0;
}
}
void insertPerson(HashTable *table, Person person) {
int index = hash(person.name);
Bucket *bucket = &table->buckets[index];
if (bucket->data == NULL) {
bucket->data = (Person*) malloc(sizeof(Person));
bucket->size = 1;
memcpy(bucket->data, &person, sizeof(Person));
} else {
bucket->size++;
bucket->data = (Person*) realloc(bucket->data, bucket->size * sizeof(Person));
memcpy(&bucket->data[bucket->size-1], &person, sizeof(Person));
}
}
Person *searchPerson(HashTable *table, char *name) {
int index = hash(name);
Bucket *bucket = &table->buckets[index];
for (int i = 0; i < bucket->size; i++) {
if (strcmp(bucket->data[i].name, name) == 0) {
return &bucket->data[i];
}
}
return NULL;
}
int main() {
HashTable table;
initHashTable(&table);
Person person1 = {"Alice", "1234567890"};
Person person2 = {"Bob", "2345678901"};
Person person3 = {"Charlie", "3456789012"};
insertPerson(&table, person1);
insertPerson(&table, person2);
insertPerson(&table, person3);
Person *result = searchPerson(&table, "Bob");
if (result != NULL) {
printf("Found person: %s, phone: %s\n", result->name, result->phone);
} else {
printf("Person not found\n");
}
return 0;
}
```
这个程序使用了哈希表来存储人员及其号码,使用了一个简单的哈希函数来计算每个人的索引。insertPerson函数将每个人插入到哈希表中,如果哈希表中已经有相同的名字,则将人员添加到该桶的末尾。searchPerson函数可以搜索哈希表中的任意一个人,如果找到了,则返回该人的指针,否则返回NULL。
阅读全文
相关推荐
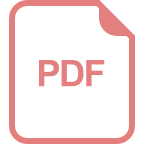
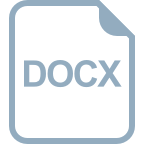
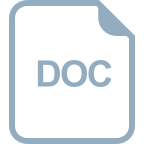
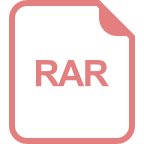
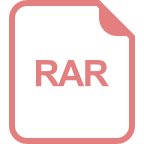
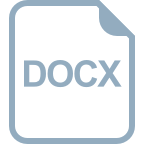
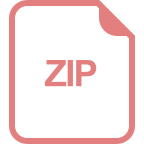
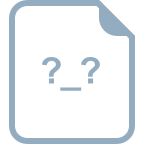
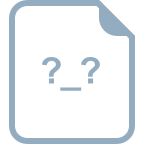
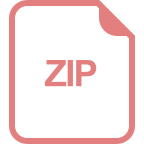
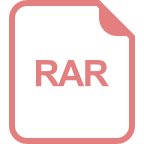
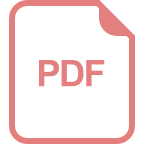
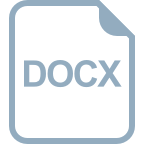
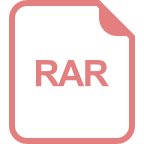